Spring / Spring interview questions
Spring is an open source framework that provides comprehensive infrastructure support for developing Java applications. It provides libraries of classes which make it easier to develop tasks such as transaction management, database integration, and web applications and create the help to address the complexity of the development of enterprise application.
Spring is a light weight inversion of control and aspect-oriented container framework.
- Lightweight. Spring is lightweight when it comes to API size and transparency. The basic version of spring framework is around 1MB and the processing overhead is also very minimal.
- Inversion of control (IoC). Loose coupling is achieved in spring using the technique Inversion of Control. The objects give their dependencies instead of creating or looking for dependent objects.
- Framework. Spring provides most of the core functionality and features, leaving rest of the coding to the developer.
- Aspect oriented (AOP). Spring supports Aspect-oriented programming and enables cohesive development by separating application business logic from system services.
- Spring is a light weight container in comparison to J2EE containers.
- Spring has layered architecture which means you require to work on the layer you wanted to enhance and leave the rest of the layers to Spring framework.
- Spring helps creating loosely coupled applications by using DI (Dependency Injection).
- Spring has Predefined Templates.
- Spring is POJO-based, non-invasive framework which allows usage of its components. POJO enables continuous integration and testability.
- Spring is independent of any Application servers.
- Spring supports data access techniques such as DAO, JDBC, JDO, Hibernate, IBATIS, etc.
- Application code is much easier to test using Junit and other unit test frameworks.
- Spring support declarative transaction management, security and logging service like AOP.
- Spring supports easy integration with third party tools and technologies like Hadoop, UI frameworks.
Below are the modules of the Spring framework.
- Core Container module.
- Application context module.
- AOP (Aspect Oriented Programming).
- JDBC abstraction and DAO module.
- ORM integration module (Object/Relational).
- Web module.
- Servlet module.
- Struts module.
- MVC framework module.
- Expression Language module.
- OXM module.
- Java Messaging Service(JMS) module.
- and Transaction module.
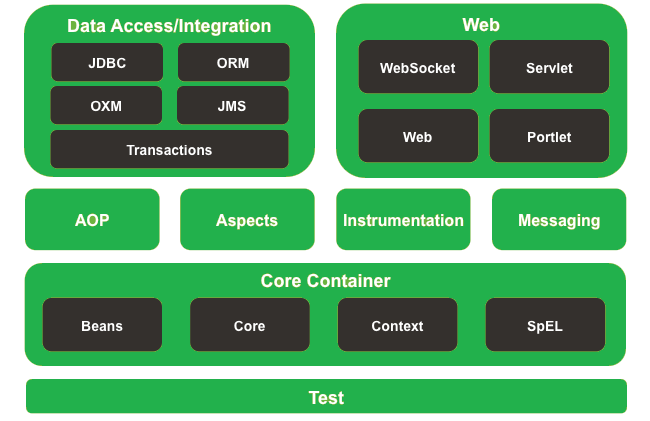
When a bean is instantiated, it may be required to perform some initialization to get it into a usable state. Similarly, when the bean is no longer required and is removed from the container, some cleanup may be required.
Spring bean factory is responsible for managing the life cycle of a Spring bean created through spring container. The life cycle of a bean consist of call back methods that can be broadly categorized into 2 groups:
- Post initialization call back methods.
- Pre destruction call back methods.
Spring framework also provides the following 4 ways to control the life cycle events of a bean:
- InitializingBean and DisposableBean callback interfaces.
- Other Aware interfaces for specific behavior.
- Custom init() and destroy() methods in bean configuration file.
- @PostConstruct and @PreDestroy annotations.
For example, Below configured beanCustomInit() and beanCustomDestroy() methods of the bean are the examples of life cycle method of Employee Bean.
<beans> <bean id="empBean" class="net.javapedia.spring.EmployeeBean" init-method="beanCustomInit" destroy-method="beanCustomDestroy"></bean> </beans>
Both refers to the scope of a beans which defines their existence on the application.
Singleton
single object instance per Spring IOC container.
Prototype
Spring IoC container creates new bean instance of the object every time a request for that specific bean is made.
There are 2 kinds of Dependency Injection Spring supports.
Setter Injection: Setter-based DI accomplished by calling setter methods on the beans after invoking a no-argument constructor or no-argument static factory method to instantiate your bean.
Constructor Injection: Constructor-based DI that works invoking a constructor with a number of arguments, each representing a dependency.
Bean Factory is like a factory class that contains collections of beans. The Bean Factory holds bean definition of multiple beans within itself and then instantiates the bean when asked by client.
Bean Factory is an actual representation of the Spring IOC container that is responsible for containing and managing the configured beans.
Use the prototype scope for all beans that are stateful and the singleton scope for stateless beans.
There are two types of dependency Injection:
Constructor Injection
Dependencies are provided as a constructor parameter.
Setter Injection
Dependencies are assigned through setter method.
Interface Injection
Injection is done through an interface and not supported in spring framework.
With ApplicationContext more than one config files are possible while only one config file or .xml file is possible with BeanFactory.
ApplicationContext support internationalization messages, application life-cycle events, validation and many enterprise services like JNDI access, EJB integration etc. while BeanFactory doesn't support any of these.
Spring supports two types of transaction management:
a. Programmatic transaction management
b. Declarative transaction management.
Programmatic transaction management can be considered for applications with less volume transaction operations and declarative transaction management is preferred for application involving large scale transactions.
Bean wiring means creating associations between application components i.e. beans within the spring container.
There are 2 types of IoC containers:
-
Bean Factory container: Simplest container providing basic support for DI. The BeanFactory is usually preferred where the resources are limited like mobile devices or applet based applications.
-
Spring ApplicationContext Container: This container adds more enterprise-specific functionality such as the ability to resolve textual messages from a properties file and the ability to publish application events to interested event listeners.
Enterprise applications have some common cross-cutting concerns that will be applied to the different types of objects and application modules such as logging, transaction management, data validation and authentication.
In object-oriented Programming, modularity of application is achieved by classes whereas in AOP application modularity is achieved by Aspects and they are configured across methods.
AOP takes out the direct dependency of cross-cutting tasks from classes which is not possible in normal object-oriented programming. For example, we can have a separate class for logging but again the classes will have to call these methods for logging the data.
There are 5 auto wiring modes in spring framework.
- no: autowiring is OFF. You have to explicitly set the dependencies using tags in bean definitions; default option for bean wiring in spring framework.
- byName: This option enables the dependency injection identified by bean names. When autowiring a property in the bean, the property name is used for searching a matching bean definition in the configuration file. If such bean is found, it is injected in the property. If no such bean is found, an error is raised.
- byType: This option enables the dependency injection where bean is identified by its type. When autowiring a property in the bean, property's class type is used for searching a matching bean definition in the configuration file. If such bean is found, it is injected in the property. If no such bean is found, an error is raised.
- constructor: Autowiring by constructor is similar to byType, however, it applies to constructor arguments. In autowire enabled bean, the beans are identified by the class type of constructor arguments, and then do an autowire by type on all constructor arguments. Note that if there isn't exactly one bean of the constructor argument type in the container, a fatal error is raised.
- autodetect: Autowiring by autodetecting uses either of two modes: constructor or byType. Spring attempts to match valid constructor with arguments, if found the constructor mode is chosen. If there is no constructor defined in bean or explicit default no-args constructor is present, the autowire byType mode is chosen.
To enable annotation based wiring, @Autowired, org.springframework.beans .factory.annotation.
AutowiredAnnotation BeanPostProcessor need to be registered and it could be achieved in 2 ways.
- Include
<context:annotation-config>
in bean configuration file.<beans> ... <context:annotation-config /> ... </beans>
- Include AutowiredAnnotation BeanPostProcessor directly in bean configuration file.
<beans> <bean class="org.springframework.beans.factory.annotation.AutowiredAnnotationBeanPostProcessor"/> </beans>
Dependency Injection is an aspect of Inversion of Control (IoC) and a general concept expressed in many different ways. As this concept suggests you do not create your objects but describe how it should be created. You don't directly associate your components and services together in code but describe the mapping and association between the services and components in a configuration file. A container (the IOC container) then holds the responsibility for hooking it all up based on the definition.
Use constructor-based DI for dependencies that are required (mandatory) and setters for dependencies that are optional.
The spring annotation is a library that facilitates the use of annotations to configure your application using spring framework. Example spring annotations include @Required, @Autowired etc.
Spring instantiate all the beans at startup by default. But sometimes, some beans are not required to be initialized during startup, rather we want them to be initialized in later stages of the application on demand. For such beans, we include lazy-init="true" while configuring beans.
<bean lazy-init="true"> <!-- this bean will be lazily-instantiated... --> </bean>
Setter injection is flexible than constructor injection because you must remember the type and order of constructor parameter. Also, constructor injection is generally used to inject the mandatory dependency, while setter can be used to inject optional dependency.
Using factory your code is still actually responsible for creating objects. By DI you delegate that responsibility to another class or a framework, which is separate from your code.
Use of dependency injection results in loosely coupled design but the use of factory pattern create a tight coupling between factory and classes.
A disadvantage of Dependency injection is that you need a container and configuration to inject the dependency, which is not required in case of factory design pattern.
Singleton is the default scope.
- Download Spring and its dependency jars from Spring site. You may also configure spring dependency using Gradle or maven.
- Create application context XML to define beans and dependencies.
- Integrate application context xml with web.xml.
- Deploy and run the application.
Using constructor-arg
property, we can pass reference to another bean or a value to constructor arguments. index attribute may be used to specify order of arguments.
<bean id = "user" class = "net.javapedia.User"> <constructor-arg ref = "fName"/> <constructor-arg value = "Miller"/> </bean>
public class User { String fName; String lName; User (String fName, String lName) { this.fName = fName; this.lName = lName; } }
Spring uses runtime polymorphism and encourages association (HAS-A relationship) rather than inheritance.
It depends actually. When spring creates a JDK dynamic proxy, the final class will work. When Spring create a CGLIB proxy and your class has at least one public method, then the class cannot be final.
String class is a final class that is widely used as spring bean.
Spring will create a CGLIB proxy if one of the following statements is true.
- aop:config has proxy-target-classes set to true.
- Any other namespace configurations (for example, transaction-management) also have proxy-target-classes set to true.
- Your class does not implement an interface.
Refer the previous question and answer. If all of the statements are not true, spring will create JDK dynamic proxy.
Yes, DTD or XSD is mandatory for Spring bean xml documents. spring-beans.jar file has the dtd (document type definition) file spring-beans.dtd under the package org.springframework.beans.factory.xml.
JDK Dynamic proxy can only proxy by interface while CGLIB (and javassist) can create a proxy by subclassing.
In JDK dynamic proxy, your target class need to implement an interface, which is then implemented by the proxy class. In CGLIB the proxy becomes a subclass of the target class so there is no need for interfaces.
JDK Dynamic proxy uses JDK reflection API. CGLib (Code Generation Library) is built on top of ASM, generates proxy extending the spring bean and adds bean behavior in the proxy methods.
Spring does not allow injecting to public static non-final fields. So the workaround will be changing to private modifier.
Another workaround will be to create a non static setter to assign the injected value for the static variable.
@Value("${my.name}") public void setName(String privateName) { ThisClass.name = privateName; }
Yes.
No. Prototype beans are stateful however if it is managed by multiple threads, then steps must be taken to ensure it is thread safe.
Yes. Each request will get its own bean so thread safety is guaranteed.
Singleton scope is preferred. DAO, service and controllers do not have to maintain its state.
JavaBeans are Java classes which adhere to certain coding conventions. Java bean classes will,
- have public default (no argument) constructors,
- have accessor (getter and setter) methods.
- implement java.io.Serializable.
Spring bean is basically an object managed by Spring. It is an object that is instantiated, configured and managed by a Spring Framework container. Spring beans are defined in Spring configuration files.
Google Guice framework.
- Generate the WAR artifact from the Spring boot project by executing the Maven/Gradle build process.
- Deploy the generated WAR into any server that has a web-container. For example, if you are using TOMCAT server, copy the WAR to the webapp folder under the tomcat folder. Tomcat auto-detects any changes under webapp folder and start deploying right away (Hot deployment).
As per the Spring-boot reference documentation, an actuator is a manufacturing term that refers to a mechanical device for moving or controlling something. Actuators can generate a large amount of motion from a small change.
You can use the ApplicationContext.getBean(String name, Object ... params)
method, which allows for specifying explicit constructor arguments / factory method arguments, overriding the specified default arguments (if any) in the bean definition.
For example, the Employee object needs 2 constructor args (id and name) to instantiate.
@Component public class Employee { private int id; private String name; public Employee(int id, String name) { this.id = id; this.name = name; } }
To create the bean, use the below code.
Integer id = 102; String name = "javapedia.net"; Employee emp = (Employee)applicationContext.getBean("employee", id, name);
@ControllerAdvice
annotation manages the exceptions in Spring Boot Application.It is an interceptor of exceptions thrown by methods annotated with @RequestMapping.
@ControllerAdvice is a specialization of the @Component annotation that allows to handle exceptions across the entire application in one global handling component.
ResponseEntityExceptionHandler
is the base class that provides centralized exception handling.