Spring / Spring Integration
Spring provides a custom JavaServer Faces VariableResolver implementation, known as DelegatingVariableResolver, extends the standard Java Server Faces managed beans mechanism which enables using JSF and Spring together.
JSF and Spring do share some of the same features, most noticeably in the area of IOC services. By declaring JSF managed-beans in the faces-config.xml configuration file, we may allow the FacesServlet to instantiate that bean at startup. Your JSF pages have access to these beans and all of their properties.We can integrate JSF and Spring in two ways:
- DelegatingVariableResolver: Spring comes with a JSF variable resolver that lets you use JSF and Spring together.
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE beans PUBLIC "-//SPRING//DTD BEAN//EN" "http://www.springframework.org/dtd/spring-beans.dtd"> <faces-config> <application> <variable-resolver> org.springframework.web.jsf.DelegatingVariableResolver </variable-resolver> </application> </faces-config>
- FacesContextUtils: custom VariableResolver works well when mapping one's properties to beans in faces-config.xml, but at times one may need to grab a bean explicitly. The FacesContextUtils class makes this easy. It is similar to WebApplicationContextUtils, except that it takes a FacesContext parameter rather than a ServletContext parameter.
ApplicationContext ctx = FacesContextUtils.getWebApplicationContext(FacesContext.getCurrentInstance());
Spring and JSF integration is useful when an event handler have to explicitly invoke the bean factory to create beans on demand, such as a bean that encapsulates the business logic to be performed when a submit button is pressed or based on any other UI events.
@EnableIntegration annotation allows the registration of Spring Integration infrastructure beans.
Spring 3.0 introduced TaskScheduler for scheduling jobs by enabling Api support for support for Timer (Jdk) and Quartz.
TaskScheduler defines the below API.
ScheduledFuture scheduleAtFixedRate(Runnable task, long period); ScheduledFuture scheduleWithFixedDelay(Runnable task, long delay);
Scheduling can be done with xml configuration as well as using annotations.
A Message Channel represents the "pipe" of a pipes-and-filters architecture. Producers send Messages to a channel, and consumers receive Messages from a channel. The Message Channel, therefore, decouples the messaging components and also provides a convenient point for interception and monitoring of Messages.

Message is a generic wrapper for any Java object combined with metadata used by the framework while handling that object. It consists of a payload and headers. The payload can be of any type and the headers hold commonly required information such as id, timestamp, correlation id, and return address.
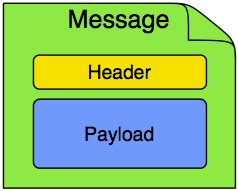
Headers hold key-value pairs.
The Endpoints primary role is to connect the application code to the messaging framework and to do so in a non-invasive manner.
Spring integration achieve the following goals.
- provides a simple model for implementing complex enterprise integration solutions.
- facilitates asynchronous, message-driven behavior within a spring application.
- promotes intuitive, incremental adoption for existing Spring users.
Transformer is responsible for converting a MessageÂs content or structure and returning the modified Message. One common type of transformer is one that converts the payload of the Message from one format to another (for example, from XML to JSON).
A Message Filter determines whether a Message should be passed to an output channel at all by evaluating a boolean test method.
A Message Router is responsible for deciding what channel(s) should receive the Message next (if any).
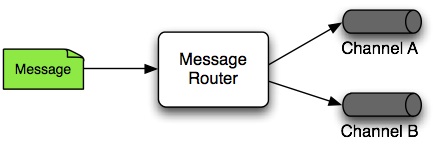
A Splitter accepts a Message from its input channel, split that Message into multiple Messages, and then send each of those to its output channel. This is typically used for dividing a "composite" payload object into a group of Messages containing the sub-divided payloads.
Aggregator is a type of Message Endpoint that receives multiple Messages and combines them into a single Message.
Service Activator is a generic endpoint for connecting a service instance to the messaging system. The input Message Channel must be configured, and if the service method to be invoked is capable of returning a value, an output Message Channel may also be provided optionally.
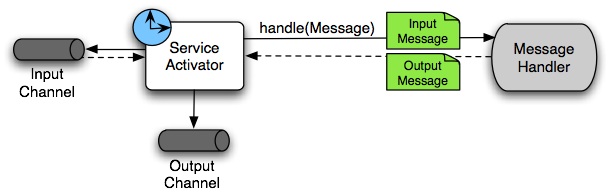
Aggregator is a type of Message Endpoint that receives multiple Messages and combines them into a single Message.
A Channel Adapter is an endpoint that connects a Message Channel to some other system or transport. Channel Adapters may be either inbound or outbound. Typically, the Channel Adapter will do some mapping between the Message and whatever object received from is received-from or sent to the other system (File, HTTP Request, JMS Message, etc).

Broadly there are 2 classifications.
- Pollable channel,
- point-to-point channel (only one receiver of a message in the channel),
- requires a quieue to hold the message and queue will have designated capacity,
- buffer its messsage.
- and Subscribable channel.
- does not buffer messages,
- allows multiple subscribers (consumers) to regisiter for its messages,
- Usually used for "event" based messaging.
Pipe and Filter pattern use components and connectors in which components, also called filters, apply local transformations to their input streams and often do their computing incrementally so that output begins before all input are consumed; Connectors, also called pipes, serve as conduits for the streams, transmitting outputs of one filter as input of another filter.
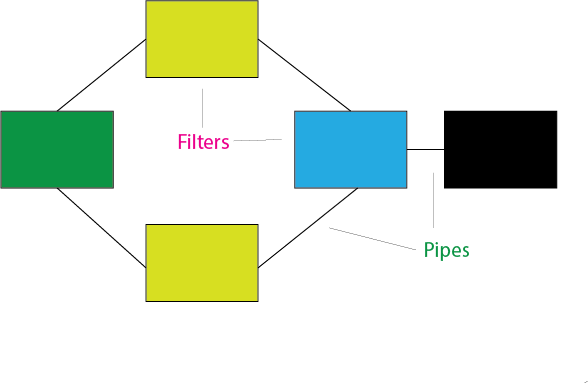
Filters do not its state. This pattern is suitable for applications that require a defined series of independent computations to be performed on data.
Apache Shiro and Apache Camel.
In synchronous communication, the sender blocks while the message is sent to the messaging system using the same thread. If an exception is raised, it is thrown reaching the application.
In asynchronous communication, Spring Integration handles exceptions by publishing them to message channels. If an exception is raised, it will not reach the application.
Error channel handles this by wrapping the exception thrown into a MessagingException, and becomes the payload of a new message.