Spring / Spring boot convert HTTP Request JSON To XML using Jackson
In this tutorial, we will discuss how to convert JSON Request Payload to XML. As this page scope is limited to JSON to XML conversion with minimal coding, no logic is covered. The controller endpoint receives a JSON Request which the controller method converts to XML and returns it to the client. Please note that the JSON structure is predefined and it works only with a particular Java model class.
Input Json:
{ "id": 1, "name": "javapedia.net", "skillSet": [ { "skillName": "Java", "category": "server programming" }, { "skillName": "Angular", "category": "frontend programming" }, { "name": "mongodb", "category": "database" } ] }
Output XML:
<RequestModel> <id>1</id> <name>javapedia.net</name> <skillSet> <skillSet> <skillName>Java</skillName> <category>server programming</category> </skillSet> <skillSet> <skillName>Angular</skillName> <category>frontend programming</category> </skillSet> <skillSet> <skillName/> <category>database</category> </skillSet> </skillSet> </RequestModel>
We add the Jackson-xml dependency in the classpath and also set produces
attribute property to "application/xml" and the conversion happens implicitly by Spring Boot Http Mession Converter.
pom.xml snippet:
<dependencies> <dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-configuration-processor</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> <scope>provided</scope> </dependency> </dependencies>
Controller Method:
@Controller public class JSONToXMLMappingController { @PostMapping(path = "/mapJsontoXML", consumes = MediaType.APPLICATION_JSON_VALUE, produces = MediaType.APPLICATION_XML_VALUE) public @ResponseBody RequestModel mapJsonToXML(@RequestBody final RequestModel request) { return request; } }
The controller method specifies that this method consumes JSON and produces XML.
Testing the endpoint using postman tool:
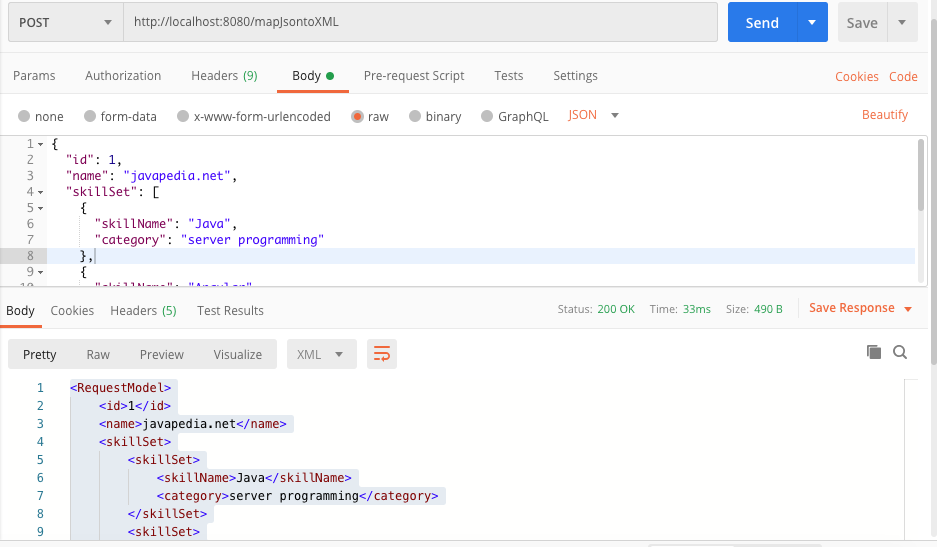
The complete project is available in Github.