Spring / Running Angular Application using JavaFX
Requisite:
- Visual studio code,
- Intellij IDE or Eclipse.
Create a new Angular application in Visual Studio Code using "ng new DemoProj" command.
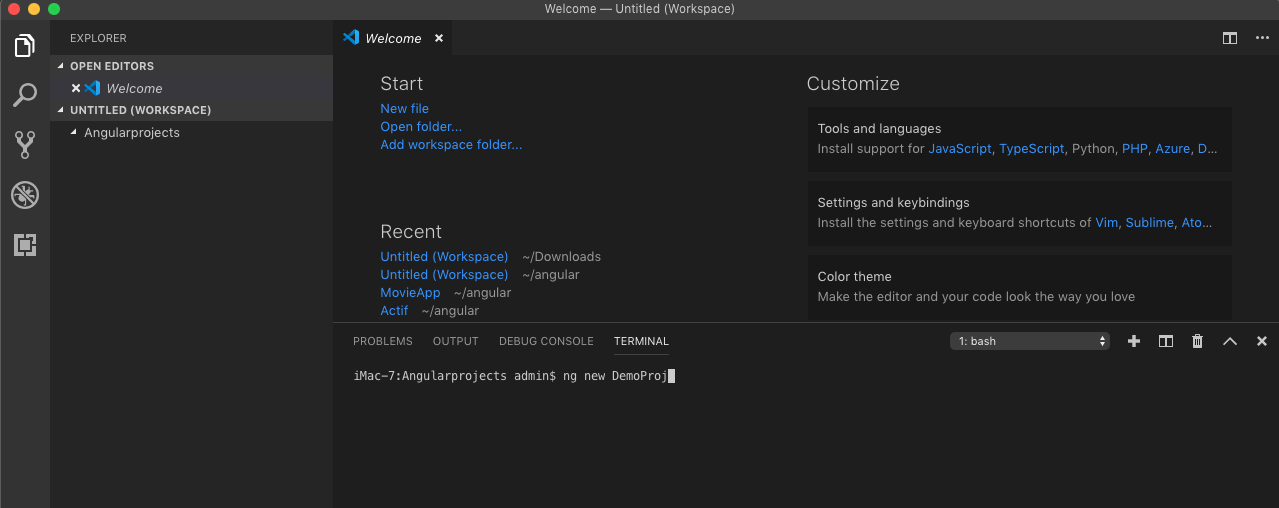
Edit app.component.html under src/app folder to have some message, for example, "Angular project loaded from JavaFx." as shown below in the picture.
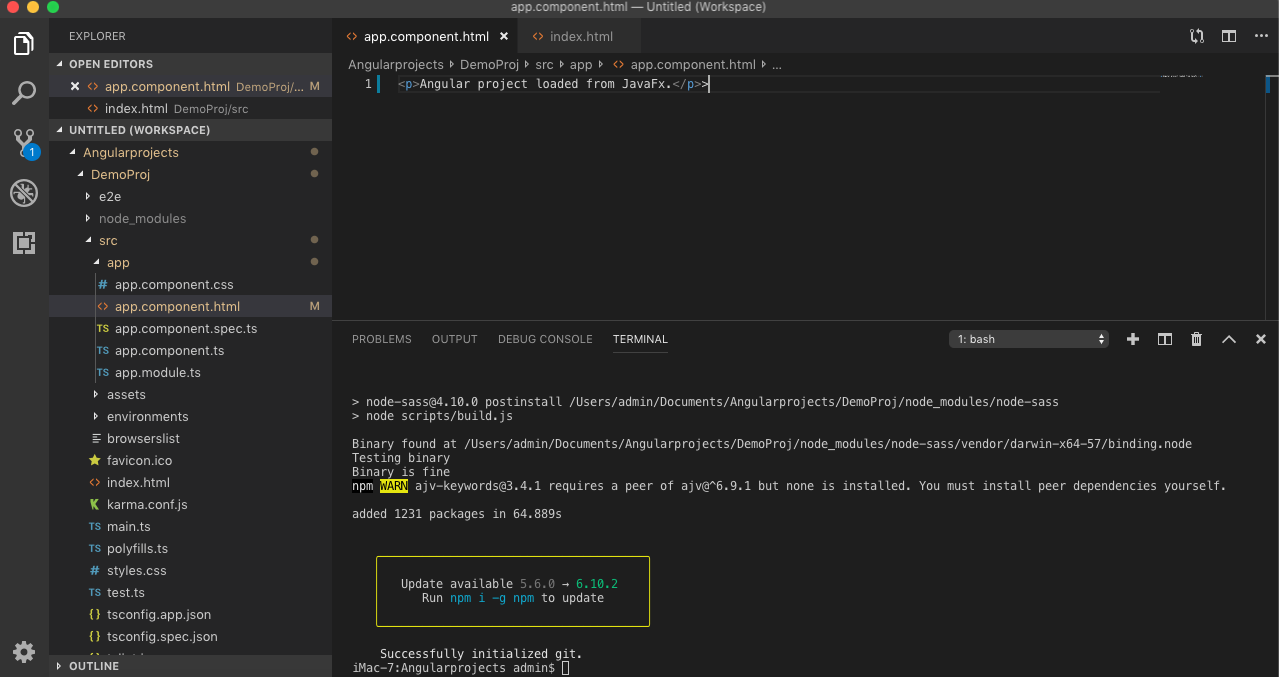
Save the changes and build your application using ng build --aot command.
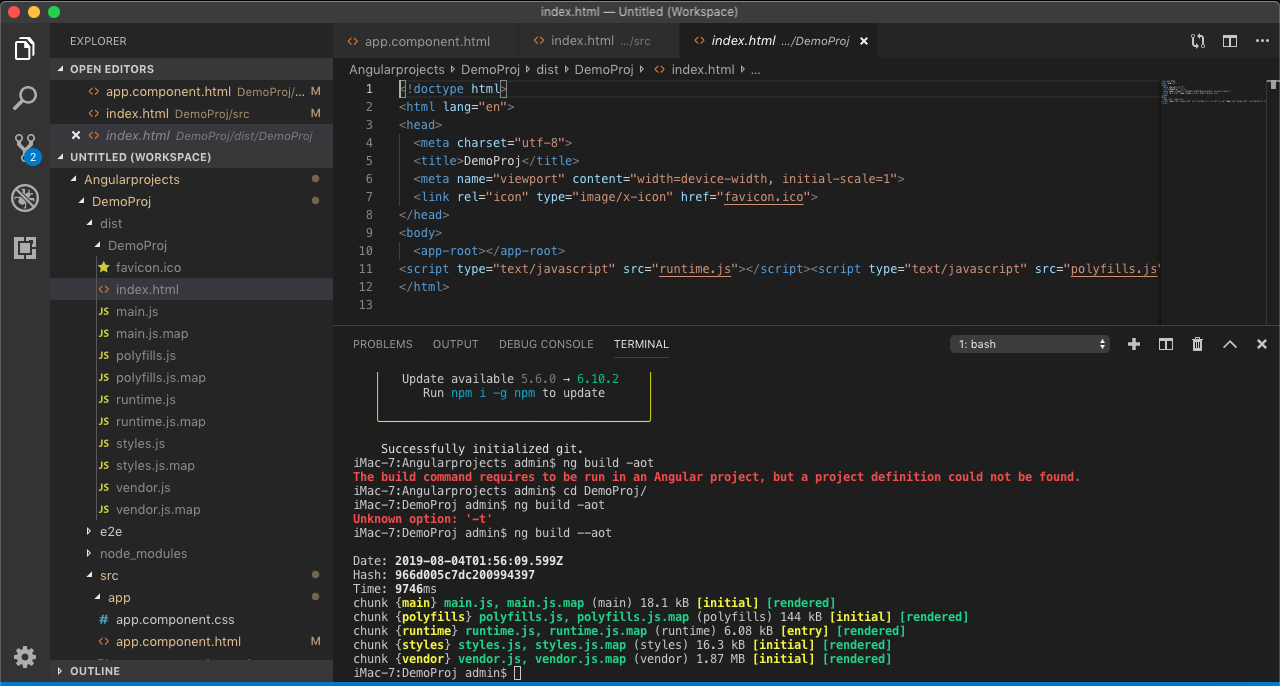
ng build command creates/update the dist folder with build artifacts.
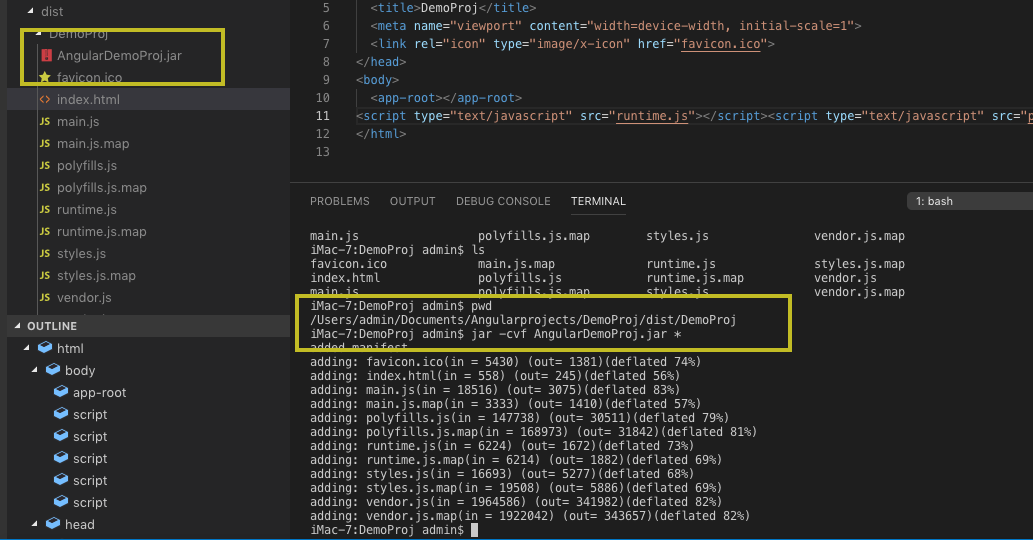
Navigate to dist/DemoProj and create the jar by running jar -cvf AngularDemoProj.jar *
command.
Creating JavaFX project.
Create new JavaFx project in Intellij IDEA IDE as shown in the picture.
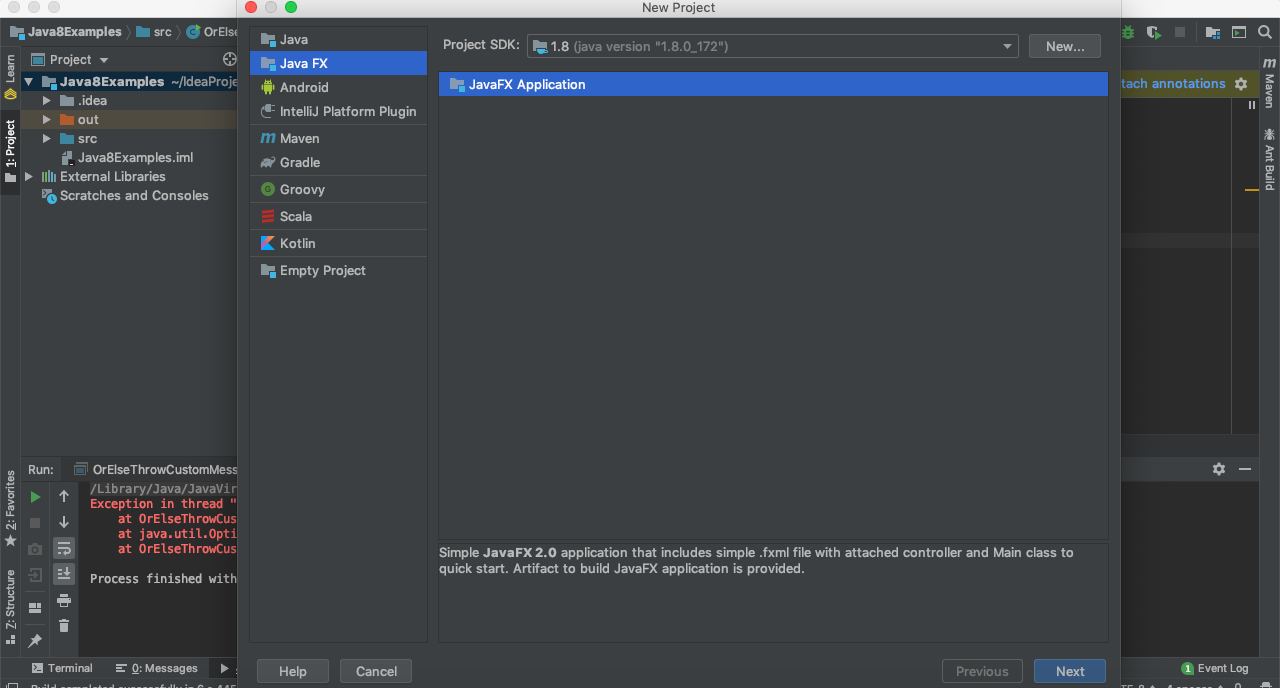
Setup the project name as, for example, JavaFxloadAngular.
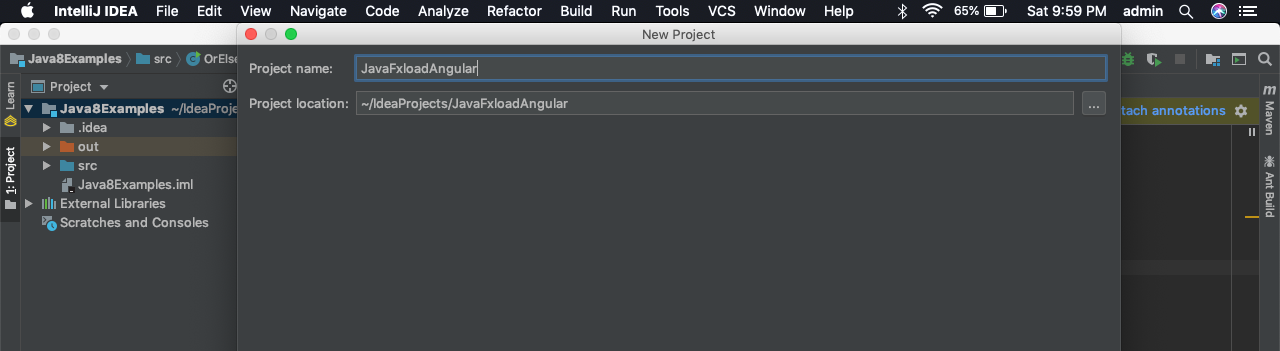
Add the AngularDemoProj.jar as dependency to the project as shown below. In Intellij IDE open the project structure, navigate to modules and click on Plus sign to add jars.
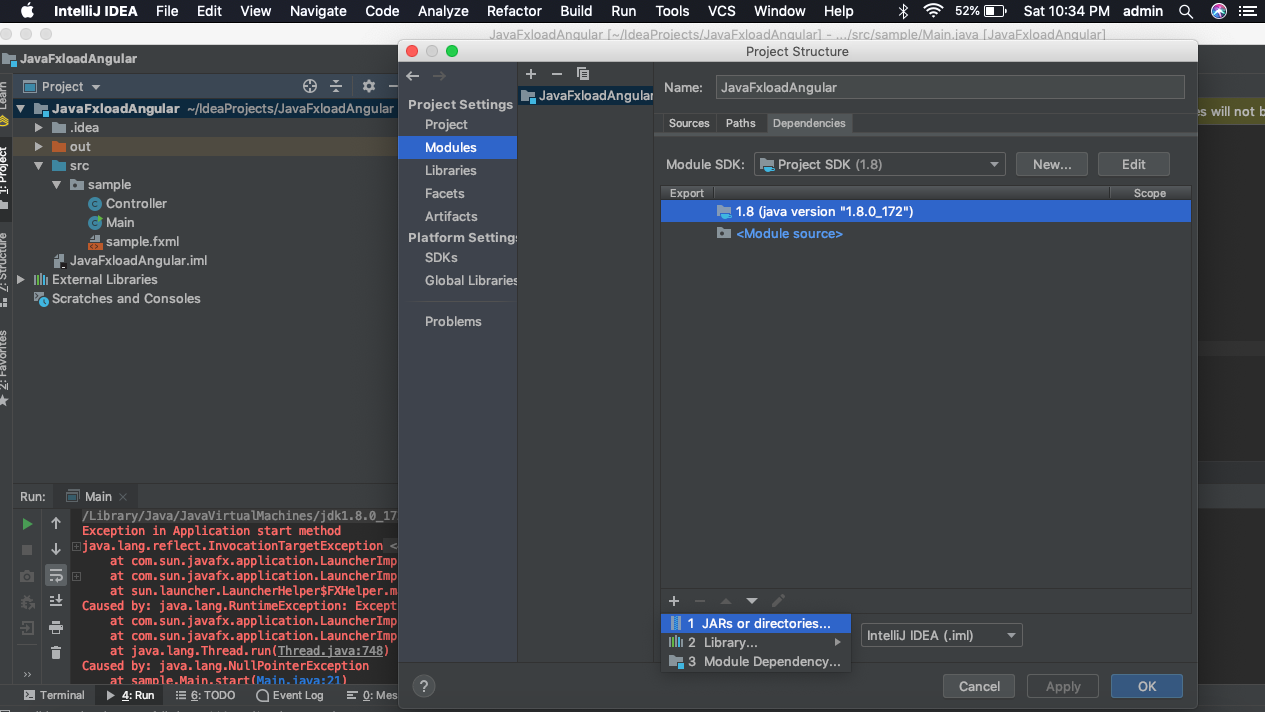
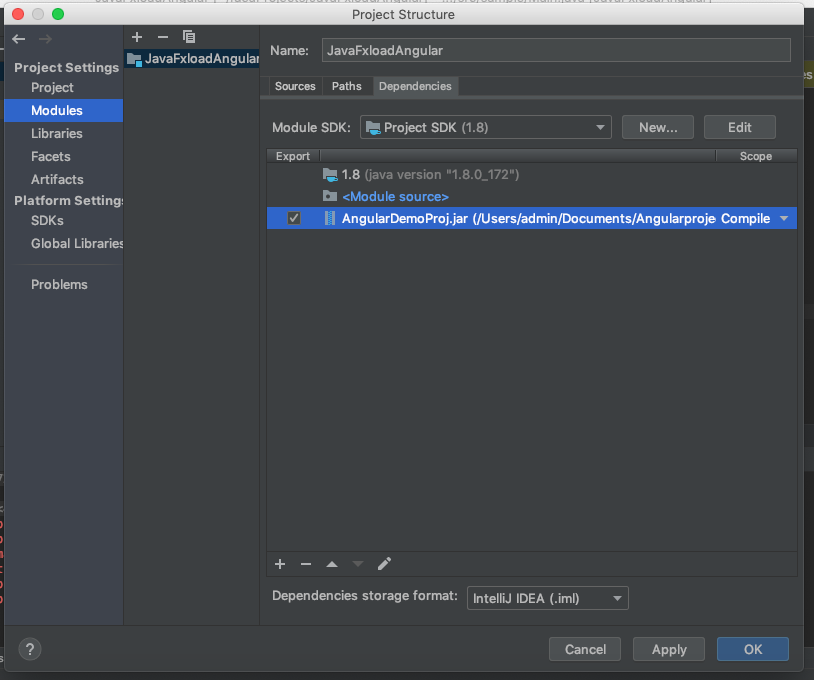
Create a sample application "Main" that creates Webview and load the index.html from the jar that we added. Copy and paste the code included here.
package sample; import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.web.WebEngine; import javafx.scene.web.WebView; import javafx.stage.Stage; public class Main extends Application { @Override public void start(Stage primaryStage) throws Exception{ Parent root = FXMLLoader.load(getClass().getResource("sample.fxml")); primaryStage.setTitle("Demo"); WebView browser = new WebView(); WebEngine webEngine = browser.getEngine(); ClassLoader classLoader = getClass().getClassLoader(); String s = classLoader.getResource("index.html").toExternalForm(); webEngine.load(s); Scene scene = new Scene(browser); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
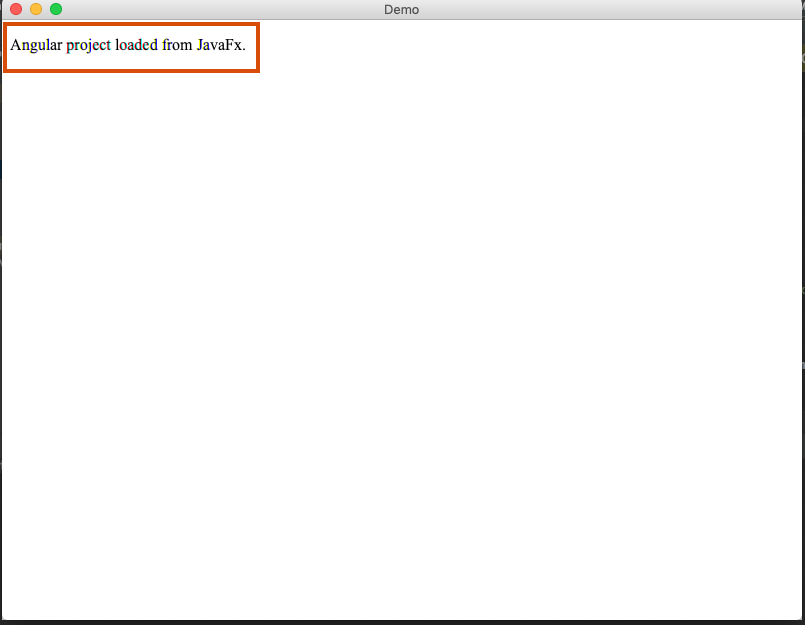
This is a basic example that shows how to load the angular project using JavaFX. JavaFX can communicate to the angular application by passing params and also sending events.
Thanks for looking at this topic. Kindly share your suggestions/feedback in the below suggestion box.