Java / OOPS Concepts and its implementation in Java
Object Oriented programming is a type of programming paradigm where programs are treated as real-world objects. OOPS correlates a computer program into a real-world object.
A real-world object, for example, a car, has properties and its associated behaviors. Car has wheels as property and its associated behavior is rotate, flat tire etc. Similarly, a computer program is considered to have data (property) and code or logic that manipulate that data (behavior).
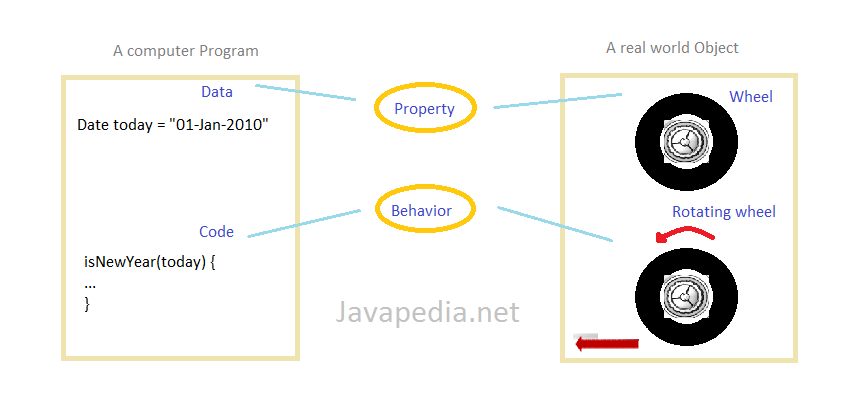
The below are the principles that attributes to the OOP behavior.
- Inheritance
- Abstraction
- Encapsulation
- Polymorphism
- Aggregation
- Composition
- Association
In object oriented programming world, the open-closed principle states that the software components classes, methods etc should be open for extension but closed for modification. A class can allow its behavior to be extended without modifying its own behavior.
Object oriented language supports all the features/principles of OOP whereas object-based languages do not support all the features.
e.g. Javascript does not support inheritance and polymorphism.
C++, Java are examples of object-oriented and Javascript and VB are object-based.
A class is a blueprint/prototype or a template for creating different objects which define its properties and behaviors.
Java class objects exhibit the properties and behaviors defined by its class.
A class provides fields and methods that describe the behavior of an object.
The cast in the below picture represents the class and the impression on the sand are the objects.
"Object" refers to an instance of a class where the object can contain any combination of variables, functions.
A variable holds or stores some data; one variable might be a color of car, another variable might be model.
The diamond problem (a.k.a deadly diamond of death) refers to an ambiguity that brews due to allowing multiple inheritance.
In Java, multiple inheritance is not allowed for classes and permitted only for interfaces to eliminates this serious issue.
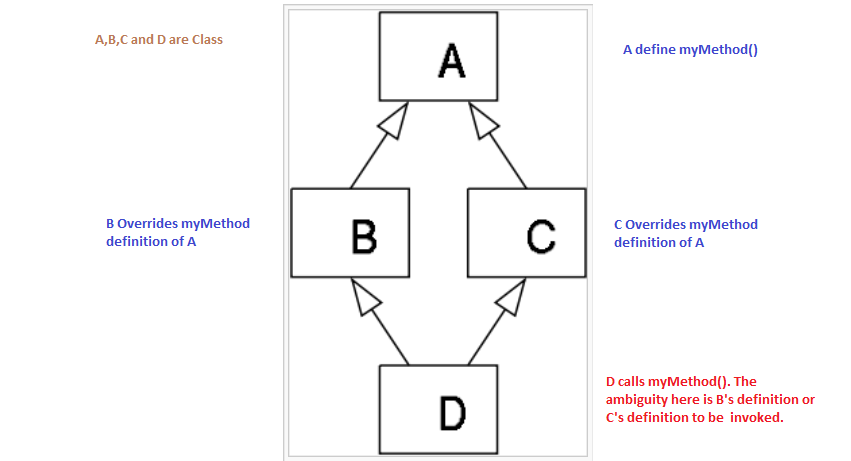
SOLID principles provide the specification for the design of a class to ensure high quality.
There are five principles as each letter in SOLID represents one.
The Single Responsibility Principle.
Every class should only serve a single purpose and that responsibility be encapsulated at the same class. For e.g. creating a CAR Class should define the properties that a car would have and along with its functions. It does not have to have Driver Class who drives it.
The Open Closed Principle.
The Open-Closed Principle (OCP) states that classes should be open for extension but be closed for modification.
The Liskov Substitution Principle.
An extension of the Open Close Principle and ensures that new derived classes(child) are extending the base class(parent) without altering its behavior.
The Interface Segregation Principle.
The Interface implementing class should not be forced to implement methods that it don't use. If it forces then it is not compliant to Interface segregation Principle and this interface referred as fat interface. The Fat interface could be fragmented further to be in align with Interface Segregation principle.
The Dependency Inversion Principle.
The principle states:
A. High-level modules should not depend on low-level modules. Both should depend on abstractions.
B. Abstractions should not depend on details. Details should depend on abstractions.
Operator overloading makes the code less readable and poorly maintainable. To maintain code simplicity, Java doesn't support operator overloading.
Static Binding. | Dynamic Binding. |
binding that happens at compile time. | binding that happens at run time. |
Actual object is not used for binding. | Actual object is used for binding. |
Method overloading is the best example of static binding. | Method overriding is the best example of dynamic binding. |
Private, static and final methods exhibit static binding. Because, they cannot be overridden. | Methods other than private, static and final exhibit dynamic binding. Because, they can be overridden. |
also called as early binding because binding happens during compilation. | also called as late binding because binding happens at run time. |
Yes. Compiler checks only method signature to validate if a particular method is overloaded or not. It does not check static or non-static nature of the method.
Encapsulation in Java is a concept that enforces protecting variables, functions from outside of class, in order to better manage that piece of code and having least impact or no impact on other parts of a program due to change in protected code.
You can completely encapsulate a member be it a variable or method in Java by using private keyword and you can even achieve a lesser degree of encapsulation in Java by using other access modifiers like protected or the public.
Data hiding is when the designer specifically decides to limit access to details of an implementation.
Abstraction is when you are dealing with an aggregate, for example, a Car is an abstraction of details such as a Motor, Wheels, brake, etc. Abstractions allow us to think of complex things in a simpler way.
Abstraction deals with separating interface from implementation. Abstraction in java is achieved by using interface and abstract class. Interface ensures 100% abstraction and abstract class provide 0-100% abstraction.
Encapsulation restricts access to or knowledge of internal structures of an implementation.
Encapsulation promotes access protection and data hiding.
The Liskov Substitution Principle is a concept in Object Oriented Programming that states: Functions that use pointers or references to base classes must be able to use objects of derived classes without knowing it.
Cohesion refers to what the class (or module) can do. High cohesion is preferred since it is focused on actions it can perform and limited. Lower cohesion means that the class does a great variety of actions, broad, unfocused.
Coupling refers to how related or dependent two classes/modules are toward each other. For low coupled classes, changing in one class should not affect the other.
So as per good Object analysis and design (OAD), high cohesion and low coupling is ideal.