Spring / Spring MVC Interview questions
The Spring web MVC framework facilitates model-view-controller (MVC) architecture and ready components that can be used to develop flexible and loosely coupled web applications.
The MVC pattern separates the different aspects of an application (for example, input logic, business logic, and UI logic) and also enables loose coupling between model, view and controller within the application.
Clear separation of roles. Model, controller, validator, command object, form object, DispatcherServlet, handler mapping, view resolver, etc. Each role can be fulfilled/extended by a specialized object.
Powerful and straightforward configuration of framework and application API as JavaBeans.
Reusable business code. You can use existing business objects as command or form objects instead of mirroring them in order to extend a particular framework base class.
Customizable binding and validation.
Customizable handler mapping and view resolution.
Convenient locale and theme resolution.
A JSP form tag library, introduced in Spring 2.0, makes it easier to write forms in JSP pages.
support for different response types; generate XML, JSON, Atom etc.
Flexible data binding: on a type mismatch, it is displayed as a validation error on the screen. Any plain Business POJO can be directly configured as form-backing object.
- The request will be received by DispatcherServlet as the first step.
- DispatcherServlet gets the help of HandlerMapping and maps the @Controller class associated with the given request to delegate the request.
- So request gets transferred to the @Controller, and then @Controller will process the request by executing appropriate methods and returns ModeAndView object (contains both Model data and View name) back to the DispatcherServlet.
- Now DispatcherServlet send the model object to the ViewResolver to resolve and retrieve the actual view page.
- DispatcherServlet will pass the Model object to the View page to display the result and create the Response.
- Finally DispatcherServlet sends the response back to the browser.
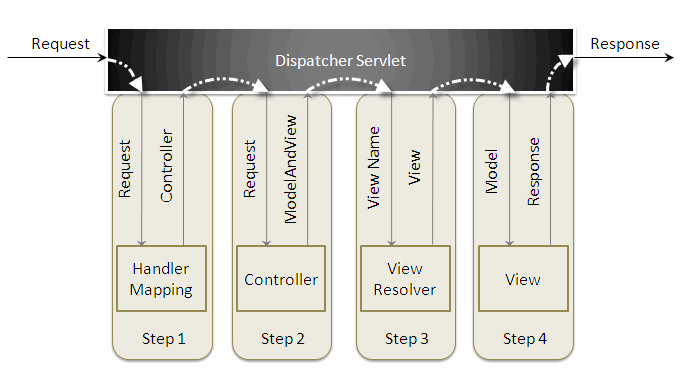
Spring web MVC framework is request-driven, flows through the central Servlet, DispatcherServlet that handles all the HTTP requests and responses. Spring's DispatcherServlet is completely integrated with the Spring IoC container so it allows you to use every feature that Spring has along with Request handling.
After receiving an HTTP request, DispatcherServlet gets helps from the HandlerMapping (configuration files) to resolve and call the appropriate Controller. The Controller takes the request and calls the appropriate service methods and set model data and then returns view name to the DispatcherServlet. The DispatcherServlet will take help from ViewResolver to resolve the defined view for the request. Once view is finalized, The DispatcherServlet passes the model data to the view which is finally rendered on the browser.
<web-app> <display-name>SpringMVC Demo App</display-name> <servlet> <servlet-name>springMVC</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springMVC</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
By default, DispatcherServlet resolves its configuration file using <servlet_name>-servlet.xml. For example, with the above web.xml file, DispatcherServlet will look for springMVC-servlet.xml file in classpath.
DispatcherServlet is the front controller in Spring MVC that intercepts every requests and then dispatches/forwards it to an appropriate controller.
ContextLoaderListener reads the spring configuration file specified using 'contextConfigLocation' in web.xml, parses it and loads the beans defined in that config file to the ApplicationContext.
<servlet> <servlet-name>springMVC</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/applicationContext.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet>
The ContextLoaderListener has purposes like,
binding the lifecycle of the ApplicationContext with that of lifecycle of the ServletContext.
creates the ApplicationContext and WebApplicationContext.
Web Application context extends Application Context which is designed to work with the standard javax.servlet.ServletContext to communicate with the container.
Beans that are instantiated in WebApplicationContext will also be able to use ServletContext if they implement ServletContextAware interface.
Yes, we can have more than one spring context files. The following are the 2 ways to configure multiple context files.
1. Specify the list of files in web.xml file using contextConfigLocation init parameter.
<servlet-name>springMVC</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value> WEB-INF/spring-core-context.xml, WEB-INF/spring-DAO.xml, WEB-INF/spring-Services.xml </param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springMVC</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
2. Import the configuration files into a existing configuration file that is already configured.
<beans> <import resource="spring-core-context.xml"/> <import resource="spring-DAO.xml"/> <import resource="spring-services.xml"/> ... //other configurations </beans>
The @Component annotation marks a java class as a bean so the component-scanning mechanism of spring loads it into the application context.
The @Repository annotation is a specialization of the @Component with similar behavior and functionality. In addition to importing the DAOs into the DI container, it also makes the unchecked exceptions (thrown from DAO methods) eligible for translation into Spring DataAccessException.
A Controller class is responsible to handle different kind of client requests based on the request mappings. We can create a controller class by using @Controller annotation. Usually it?s used with @RequestMapping annotation to define handler methods for specific URI mapping.
Spring MVC controllers are singleton by default and any controller object variable/field will be shared across all the requests and sessions.
If the object variable should not be shared across requests, one can use @Scope("request") annotation above your controller class definition to create instance per request.
avoid redirect instead use forward.
@RequestParam and @PathVariable annotations are used for accessing the values from the request.
The primary difference between @RequestParam and @PathVariable is that @RequestParam used for accessing the values of the query parameters where as @PathVariable used for accessing the values from the URI template.
Handler interceptors are required when specific functionality need to be applied to certain requests. Handler Interceptors should implement the interface HandlerInterceptor and override the below methods.
preHandle is called before the actual handler is executed;
postHandle is called after the handler is executed;
afterCompletion is called after the request is complete.
View Resolver pattern is a J2EE pattern that enables a web application to dynamically select its view technology. For example, HTML, JSP, Tapestry, JSF, XSLT etc. In this pattern, View resolver holds mapping of different views, controller return name of the view, which is then delegated to the View Resolver for selecting an appropriate view. Spring MVC framework supplies inbuilt view resolver for selecting views.
Spring provides an integrated framework for all tiers of your application.
Spring provides a very clean separation between controllers, JavaBean models, and views.
Spring Controllers are configured using IoC like any other objects. This makes them easy to test and integrated with other objects managed by Spring.
Spring MVC web tiers are typically easier to test than Struts web tiers, due to the elimination forced concrete inheritance and explicit dependence of controllers on the dispatcher servlet.
Spring MVC is entirely based on interfaces that makes it very flexible unlike Struts, which forces your Action and Form objects into concrete inheritance.
Spring MVC is truly view-agnostic. you may choose view as JSP or Velocity, XLST or other view technologies.Developers can easily implement the Spring View interface to create a custom view mechanism.
Spring provides interceptors as well as controllers, making it easy to factor behaviors common to the handling of many requests.
ModelAndView is an object that holds both the model and view. The handler returns the ModelAndView object and DispatcherServlet resolves the view using View Resolvers and View.
The View is an object which contains view name in the form of the String and model is a map to add multiple objects.
In the below example 'employeeDetails' is the view name.
ModelAndView model = new ModelAndView("employeeDetails"); model.addObject("employeeObj", new EmployeeBean(123)); model.addObject("msg", "Employee information."); return model;
Programming Tip : Get an instant remote access to your essential programming/testing work from anywhere on any device(PC/Mac/Linux/android/iOS) by accessing your programming tools online with high performance hosted citrix xendesktop with an affordable xendesktop pricing. Learn more about Hosted SharePoint, QuickBooks Cloud Hosting and Office 365 suite Enterprise E3 by visiting Apps4Rent.com.
- Controller,
- AbstractCommandController,
- SimpleFormController,
- WizardFormController,
- and MultiActionController.
MultipartResolver interface is used for uploading files; CommonsMultipartResolver and StandardServletMultipartResolver are 2 implementations provided by spring framework to facilitate file uploading. By default there are no multipart resolvers configured, to enable it define a bean named 'multipartResolver' with type as MultipartResolver in spring bean configurations.
Once configured, any multipart request will be resolved by the configured MultipartResolver and pass on a wrapped HttpServletRequest. Then it is used in a controller class to get the file and process it.
Spring MVC Framework provides the following methods to achieve robust exception handling.
Controller Based- define the exception handler methods in controller classes and annotate these methods with @ExceptionHandler
annotation.
Global Exception Handler- Exception Handling is a cross-cutting concern and Spring provides @ControllerAdvice
annotation that we can use with any class to define our global exception handler.
HandlerExceptionResolver implementation- Spring Framework provides HandlerExceptionResolver
interface that we can implement to create global exception handler. The reason behind this additional way to define global exception handler is that Spring framework also provides default implementation classes that we can define in our spring bean configuration file to get spring framework exception handling benefits.
To handle views in Spring MVC, we need to configure InternalResourceViewResolver bean in spring XML where we need to define prefix and suffix of the views name.
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/pages/"/> <property name="suffix" value=".jsp"/> </bean>
To create a Controller in Spring MVC, create a class and annotate it with @Controller and @RequestMapping annotations. @Controller declares this class as a controller and @RequestMapping defines the path mapping of request url to the controller.
Use JSTL, to retrieve values from Model as ,
${userId}.
Create a class implementing WebApplicationInitializer interface and override onStartup() method. In the method, we can register annotation based application configuration class, servlet and mappings, listener etc.
Using @EnableWebMvc, spring enables the MVC related configuration.
@EnableWebMvc annotation is applied on configuration class with @Configuration annotation.
Set the controller method return type as void and mark the method with @ResponseBody annotation.
Set the return type of the method as String and mark the method with @ResponseBody annotation.
@RequestMapping(value="/returnHelloWorld", method=GET) @ResponseBody public String returnHelloMethod() { return "Hello world!"; }
When a controller method is marked with @ResponseBody, spring deserializes the returned value of the method and writes it directly to the Http Response automatically.
The return value of the method constitute the body of the HTTP response and not placed in a Model, or interpreted as a view name.
Spring automatically converts the content of the incoming request body to the parameter object when annotated with the @RequestBody annotation.
@ResponseBody @RequestMapping("/getUserInfo") public String getUserInformation(@RequestBody UserDetails user){ return user.getFirstName() + " " + user.getLastName(); }
Construct the base URI and then use path variables. If the issue persists, change the method from GET to POST or vice versa for one of the methods.
The return type of the getParamter is String and it returns null if the parameter does not exist.
Installing Java Cryptography Extension (JCE) Unlimited Strength Jurisdiction Policy Files in your java 7/8 installation is one of the ways to resolve SSL handshake failure.
Using @JsonProperty("JSON propery name") we can configure and map POJO class property name with the JSON property.
To use the servlet container configured JNDI DataSource, we need to wire it in the spring bean configuration file and then inject it to spring beans as dependency. Once done, we can use it with JdbcTemplate to perform database operations.
< bean id="dataSource" class="org.springframework.jndi.JndiObjectFactoryBean"> < property name="jndiName" value="java:comp/env/jdbc/MySQLDB"/> < /bean>
Spring framework provides LocaleResolver to support the internationalization and localization. To have your Spring MVC application support the internationalization, you will need to register two beans.
SessionLocaleResolver resolves locales by inspecting a predefined attribute in the user's session. If the session attribute does not exist, this locale resolver determines the default locale from the accept-language HTTP header.
LocaleChangeInterceptor interceptor detects if a special parameter is present in the current HTTP request. The parameter name can be customized with the paramName property of this interceptor. If such a parameter is present in the current request, this interceptor changes the user?s locale according to the parameter value.
Spring supports validations primarily into two ways.
Using JSR-303 Annotations and any reference implementation, for example, Hibernate Validator.
Using custom implementation of org.springframework.validation. Validator interface.
Spring provides MultipartResolver to handle the file upload process in web application. There are two concrete implementations included in Spring.
- CommonsMultipartResolver for Jakarta Commons FileUpload.
- StandardServletMultipartResolver for Servlet 3.0 Part API.
To define an implementation, create a bean with the id 'multipartResolver' in DispatcherServlet application context. Such a resolver gets applied to all requests handled by that DispatcherServlet. If a DispatcherServlet detects a multipart request, it will resolve it via the configured MultipartResolver and pass a wrapped HttpServletRequest. Controllers can then cast their given request to the MultipartHttpServletRequest interface, which permits access to any MultipartFiles.
A front controller is defined as a controller that handles all requests for a Web Application. DispatcherServlet servlet is the front controller in Spring MVC that intercepts every request and then dispatches requests to an appropriate controller. When a web request is sent to a Spring MVC application, dispatcher servlet first receives the request. Then it organizes the different components configured in Spring?s web application context (e.g. actual request handler controller and view resolvers) or annotations present in the controller itself, all needed to handle the request.
HandlerInterceptor interface can be used in spring mvc application to pre-handle and post-handle web requests that are handled by Spring MVC controllers. These handlers are mostly used to manipulate the model attributes returned/submitted before it is passed to the views/controllers.
A handler interceptor can be registered for particular URL mappings so that it only intercepts requests mapped to certain URLs. Each handler interceptor must implement the HandlerInterceptor interface, which contains three callback methods for you to implement: preHandle(), postHandle() and afterCompletion().
Implementing HandlerInterceptor interface forces to implement all the three methods preHandle(), postHandle() and afterCompletion() irrespective of whether it is needed or not. To avoid that, you can use HandlerInterceptorAdapter class that implements HandlerInterceptor and provides default empty implementations. Extending HandlerInterceptorAdapter class allows you to override only the method that are required.
Spring provides spring-boot-starter-web that can resolve all Spring MVC required JAR. In our project, we can include it using maven as dependency.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>1.2.2.RELEASE</version> </dependency>
Create a class implementing WebApplicationInitializer interface and implement onStartup() method. In this method we can register all the annotation based application configuration classes, servlet and its mappings, listener etc including DispatcherServlet.
public class WebAppInitializer implements WebApplicationInitializer { public void onStartup(ServletContext servletContext) throws ServletException { AnnotationConfigWebApplicationContext ctx = new AnnotationConfigWebApplicationContext(); ctx.register(ApplicationConfig.class); ctx.setServletContext(servletContext); Dynamic dynamic = servletContext.addServlet("dispatcher", new DispatcherServlet(ctx)); dynamic.addMapping("/"); dynamic.setLoadOnStartup(1); } }
Create a bean in @Configuration class for UrlBasedViewResolver. This bean has different methods like setPrefix, setSuffix and setViewClass.
@Bean public UrlBasedViewResolver setupViewResolver() { UrlBasedViewResolver resolver = new UrlBasedViewResolver(); resolver.setPrefix("/views/"); resolver.setSuffix(".jsp"); resolver.setViewClass(JstlView.class); return resolver; }
At service layer.
Spring MVC is the standard solution for MVC by Spring for your web applications and it comprises of a view(your JSP page) , a controller class (annotated with @Controller ) and a model which represents your data.
Spring Webflow is built on top of Spring MVC and differs in purpose although it inherits most of the features of Spring MVC. Spring webflow is more suited for wizard/workflow style applications where you enter data on a form and that has to pass through a series of validations and capture number of additional data on different pages before you complete your business process.
Use a BindingResult object as an argument to the validate method of a Validator inside a Controller and the BindingResult object will hold the validation errors.
validator.validate(modelObject, bindingResult); if (bindingResult.hasErrors()) { // Handle error }
It resource reference to an object factory for resources such as a JDBC DataSource, a JavaMail Session, or custom object factories configured into Tomcat or any other application server.
In Weblogic, the JNDI lookup for "dsJDBC" is just "dsJDBC" whilst in Tomcat, it accepts only the the formal format "java:comp/env/jdbc/dsJDBC".
In Tomcat, JndiLocatorSupport has a property resourceRef. When setting this true, "java:comp/env/" prefix will be prepended automatically.
Using mvc:resources tag we can specify the cache period.
<mvc:resources mapping="/resources/**" location="/WEB-INF/resources/" cache-period="31556926"/>
Also mvc:interceptor can be used to specify the cache settings.
<mvc:interceptor> <mvc:mapping path="/WEB-INF/resources/*"/> <bean id="webContentInterceptor" class="org.springframework.web.servlet.mvc.WebContentInterceptor"> <property name="cacheSeconds" value="31556926"/> <property name="useExpiresHeader" value="true"/> <property name="useCacheControlHeader" value="true"/> <property name="useCacheControlNoStore" value="true"/> </bean> </mvc:interceptor>
do extension implies "do something". This extension convention is followed from struts1.
With the web application upgrades developers might have thought to retain and continue to use the do extension for backward compatibility.
@RequestMapping annotation is used to map a URL to either an entire controller class or a particular handler method.
Spring supports suffix ".*" so. /employee is also mapped to /employee.* /employee.xml or /employee.pdf is also mapped. To completely disable the use of file extensions, you must set both of the following:
- useSuffixPatternMatching(false),
- favorPathExtension(false).
References:
stackoverflow.
docs.spring.io
Spring Web MVC is the original web framework included with the spring framework as Servlet API and Servlet containers.
On the other hand, Spring Webflux, added in Version 5.0, is a reactive-stack web framework that provides fully non-blocking, supports reactive streams backpressure for web applications. Webflux also runs on servers like Netty, Undertow, and Servlet 3.1 + containers.
A HTTP multipart request are constructed by HTTP clients to send files and data over to a HTTP Server. It is commonly used by browsers and HTTP clients to upload files to the server.
Working out which data format to return as a response is called Content Negotiation.
Spring supports a couple of conventions for selecting the content type format required. By default they are checked in this order:
- Add a path extension (suffix) in the URL. For example, http://myserver/myapp/accounts/list.json suggests that the return format is json.
- Add URL parameter. For example, the url,http://myserver/myapp/accounts/list?format=xls, here the parameter name is format and it suggest return format as xls.
- Accept HTTP header property.
The @GetMapping is a composed annotation which is nothing but the short notation of @RequestMapping(method = RequestMethod.GET)
. Both these methods support the "consumes". The consume options are such as,
consumes = "text/plain" consumes = {"text/plain", "application/*"}: