Java / Design Patterns
Design patterns are experimented, highly validated and achieved way to solve a particular design issues by various programmers/experts around the world. Design patterns are extension to code reuse.
Types of design patterns include creational, structural, and behavioral design patterns.
Observer design pattern is based on notifying the changes in the state of object to observers so that they can perform action related to the state change. An ideal example will be a stock exchange where changes in the stock price must be reflected in Views to show to public. Here stock object is subject while different views are Observers.
- Decorator design pattern used in various Java IO classes,
- Singleton pattern used in Runtime, Calendar and various other classes,
- Factory pattern used along with various Immutable classes likes Boolean e.g. Boolean.valueOf
- and Observer pattern used in Swing and other event listener frameworks.
Strategy pattern is useful for implementing set of related algorithms for example, compression algorithms, filtering strategies etc. Strategy design pattern allows you to create Context classes, that uses Strategy implementation classes for applying business rules. This pattern follows open closed design principle and quite useful in Java.
One example of Strategy pattern from JDK is a Collections.sort() method and Comparator interface, which is a strategy interface and defines strategy for comparing objects. Because of this pattern, we don't need to modify sort() method (closed for modification) to compare any object, at same time we can implement Comparator interface to define new comparing strategy (open for extension).
State design pattern is used to define and manage the state of the object, while Strategy pattern is used to define a set of interchangeable algorithms and let's client choose one of them. So Strategy pattern is a client-driven pattern while Object can manage their state itself.
Singleton pattern ensures that only one instance or object of a class exists with the application and make sure that instance is available globally so that there is no need to create new instance of course there is no way to create more than one object.
Create a public class having a private constructor. Private constructor ensures that the object cannot be created outside of the class.
Create a private static variable of the type Class itself.
Create a public static method e.g. getInstance() that returns the reference of the static variable, if the static variable is null then create a object for the class.
package org.javatutorials.designpatterns; //Step 1 public class SingletonPattern { //Step 2 private static SingletonPattern mySingletonObj; private SingletonPattern() { } public static SingletonPattern getInstance() { if (mySingletonObj == null) { mySingletonObj = new SingletonPattern(); } return mySingletonObj; } }
- Singleton class prevent creation of many objects to the class.
- Object creation of that class could be time-consuming.
- Objects that are used across the application be singleton as it is very convenient to maintain the state.
The decorator pattern (also known as Wrapper) is a design pattern that allows behavior to be added to an individual object, either statically or dynamically, without affecting or modifying the existing behavior of other objects from the same class.
The Decorator is known as a structural pattern.
Decorator pattern is another popular java design pattern question which is common because of its high usage in java.io package. BufferedReader and BufferedWriter are good example of decorator pattern in Java.
Decorator pattern is used to implement functionality on already created object, while Proxy pattern is used for controlling access to object. Another difference between Decorator and Proxy design pattern is that, Decorator doesn't create object, instead it get object in it's constructor, while Proxy actually creates objects.
Use Setter injection to provide optional dependencies of an object, while use Constructor injection to provide mandatory dependency of an object, without which it can not work.
Composite pattern is core Java design pattern, which allows you to treat both the whole and part of the object to treat in similar way. Client code that deals with the Composite or individual object doesn't differentiate on them, it is possible because Composite class also implement same interface as there individual part.
One of the good example of Composite pattern from JDK is JPanel class, which is both Component and Container. When paint() method is called on JPanel, it internally calls paint() method of individual components and let them draw themselves.
The front controller design pattern provides a single handler for all the incoming requests for a resource in an application and then dispatches the requests to the appropriate handler for that type of request. The front controller may use other helpers API to achieve the dispatching mechanism.
ActionServlet in Struts 1 framework or Dispatchers in Struts 2 implements the Front controller design pattern.
MVC (Model-View-Controller) is a J2EE design pattern that decouples data access logic and presentation from business logic. MVC is a software architecture pattern for developing web application.
Model represents the application data domain. Application business logic is contained within the model and is responsible for maintaining data.
View It represents the user interface, with which the end users communicates and the model data is presented and viewed.
The Controller reacts to the user input. It creates, populates the model and helps identify the relevant view.
There are multiple ways to write thread-safe singleton in Java.
- using double checked locking,
- by using static Singleton instance initialized during class loading.
- using Java enum to create a thread-safe singleton class.
Using Java enum to create thread-safe singleton is the most simplest way.
Both Abstract Factory and Factory design patterns are creational design pattern and is used to decouple clients from creating object they need.
The difference is Abstract Factory pattern delegates its responsibility of instantiating the object to another object by composition while the Factory Method pattern utilize inheritance and depends on a subclass to handle the object instantiation.
No. Decorator design pattern adds new behaviors without modifying the existing behaviours to an object which resembles AspectJ and not the EJB.
The Facade Pattern makes one single complex interface easier to use, using a Facade class. The Facade Pattern provides a unified interface to a set of interface in a subsystem. Facade defines a higher-level interface that makes the subsystem easier to use.
Java Design Patterns are divided into three categories: creational, structural and behavioral design patterns.
Abstract Factory, Builder, Factory method, prototype and singleton are some of the creational design patterns.
Adapter, Bridge, Composite, Decorator,Flyweight, Facade and proxy are some of the structural design patterns.
Chain of Responsibility, Iterator, Strategy, Template, Command, Observer, state and Mediator are some of the behavioral design patterns.
Builder pattern, a creational design pattern was introduced to solve some of the problems with Factory and Abstract Factory design patterns when the Object contains too many attributes.
The builder pattern is a design pattern that allows for the step-by-step creation of complex objects using the correct sequence of actions. The construction is controlled by a director object that only needs to know the type of object it is to create.
Factory pattern is one of the Creational Design Patterns.
Factory pattern is used to construct objects such that they can be decoupled from the implementing system. It states that 'define an interface for creating an object, but let the subclasses decide which class to instantiate. The Factory method lets a class defer instantiation to subclasses'.
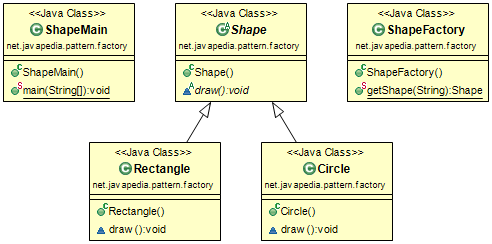
An API describes the classes, interfaces, methods that you call and use to achieve a goal while SPI describes the classes,interfaces, methods that you extend and implement to achieve a goal.
In JDBC the Driver class is part of the SPI: If you simply want to use JDBC, you don't need to use it directly, but everyone who implements a JDBC driver must implement that class.
The Connection interface is both SPI and API: You use it routinely when you use a JDBC driver and it needs to be implemented by the developer of the JDBC driver.
A service provider framework is a system in which multiple service providers implement a service, and the system makes the implementations available to its clients, decoupling them from the implementations.
There are 3 essential components of a service provider framework: a service interface, which providers implement; a provider registration API, which the system uses to register implementations, giving clients access to them; and a service access API, which clients use to obtain an instance of the service. The service access API typically allows but does not require the client to specify some criteria for choosing a provider. In the absence of such a specification, the API returns an instance of a default implementation. The service access API is the "flexible static factory" that forms the basis of the service provider framework.
An classic example of SPI is JDBC, where Connection acts as the service interface, DriverManager.registerDriver as provider registration API, DriverManager.getConnection as service access API, and Driver is the service provider interface.
In Java there is no support for default values for constructor parameters.
Telescoping constructor comes to the rescue. A class has multiple constructors, where each constructor calls a more specific constructor in the hierarchy, which has more parameters than itself, providing a default value for the extra parameters. The next constructor does the same until there is no left.
public Person(String firstName, String lastName) { this(firstName, lastName, null); } public Person(String firstName, String lastName, String description) { this(firstName, lastName, description, 0); } public Person(String firstName, String lastName, String description, int age) { this.firstName = firstName; this.lastName = lastName; this.description = description; this.age = age; }
Using JavaBeans pattern, you call a parameterless constructor to create the object and then call setter methods to set each required parameter and each optional parameter of interest as required.
private Person person1= new Person(); person1.setFirstName("Steve"); person1.setLastName("Jobs"); person1.setDescription("Apple Founder");
This is an alternative to the telescopic constructor pattern. Java beans pattern allows inconsistency and mandates mutability which is a drawback.
- JavaBean will be in inconsistent state partway through its construction.
- The JavaBeans pattern makes it impossible of making a class immutable.
- It allows inconsistency and mandates mutability.
Builder pattern combines the safety of the telescoping constructor pattern with the readability of the JavaBeans pattern.
To create the desired object, the client first calls a constructor (or static factory) with all of the required parameters and gets a builder object. Then the client calls setter-like methods on the builder object to set each optional parameter of interest. Finally, the client calls a parameter-less build method to generate the object, which is immutable.
The builder is a static member class of the class it builds.
public class BuilderPatternEx { static class Person { private String fName; private String lName; private short age; private String employerName; public static class PersonBuilder { private String fName; private String lName; private short age; private String employerName; public PersonBuilder(String fName, String lName) { this.fName = fName; this.lName = lName; } public PersonBuilder age(short age) { this.age = age; return this; } // The builder's setter methods return the builder itself so that // invocations can be chained. public PersonBuilder employerName(String employerName) { this.employerName = employerName; return this; } public Person build() { return new Person(this); } } Person(PersonBuilder p) { this.fName = p.fName; this.lName = p.lName; this.age = p.age; this.employerName = p.employerName; } @Override public String toString() { StringBuilder temp = new StringBuilder("Peron details: "); temp.append(this.fName).append(" ").append(this.lName).append(" of age ").append(this.age); return temp.toString(); } } public static void main(String[] args) { Person p = new Person.PersonBuilder("John", "Jacob").age((short) 45).employerName("Apple").build(); System.out.println(p); } }
In order to create an object, you must first create its builder. It could be a problem in some performance critical situations.
The Builder pattern is preferred for classes whose constructors or static factories would have more parameters (four or more), especially if most of those parameters are optional.
Create a constructor and throw InstantiationError when the private singleton instance reference is not null.
private Singleton() { if( Singleton.singleton != null ) { throw new InstantiationError( "Creating of this object is not allowed." ); } }
Each JVM will have its own copy of singleton object in clustered environment, so we may have to use different techniques to ensure only one instance exists across multiple JVMs in clustered environment.
Terracotta, Oracle Coherence provides an in memory replication of objects across JVMs that enables singleton view.
Cluster-aware cache providers like Swarm Cache or JBoss TreeCache create cache entries as singletons.
JBoss and Weblogic has the concept of Singleton Service where only instance runs within the cluster and all clients will look up to the same instance. WebSphere application server also supports the concept of singleton across clusters.
JMS, JGroups software allows to form a group and Applications (JVMs) can participate and JGroups will send messages to everyone in the group so that they can be in sync.
Singleton pattern gives you an Object, while later just provide static methods.
Static class provides better performance than Singleton pattern, because static methods are bonded on compile time.
Static methods cannot be overridden while we can override methods defined in a Singleton class by extending it.
Singleton classes can be lazy loaded, but static class does not have such advantages and always eagerly loaded.
If you need to maintain state than Singleton pattern is better choice than static class as it requires additional synchronization mechanism.
With Singleton, you can use Inheritance and Polymorphism to extend a base class, implement an interface and capable of providing different implementations.
singleton can be serialized to preserve its state.
java.lang.Runtime is a Singleton class in Java.
If your Singleton is not maintaining any state, and just provide global access to methods, then consider using static class, as static methods are faster than Singleton, because of static binding during compile time.
Bridge design pattern is a structural design pattern.
It states that "Decouple the abstraction from its implementation so that both can vary independently".
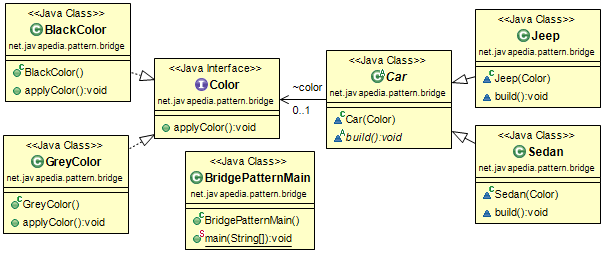
The example code is available here.
Prototype pattern is one of the creation design patterns in Java which states that "Create objects based on a template of an existing object through cloning".
Template pattern outlines an algorithm in template method and let subclass implement individual steps.
Template method is final, so that subclass cannot override and change the steps, however individual steps are declared abstract, so that child classes can implement them.
Filter pattern also known as Criteria pattern, enables developers to filter a set of objects using different criteria and chaining them in a decoupled fashion through logical operations. This is a structural pattern as it combines multiple criteria to obtain single criteria.
Proxy design pattern, a structural pattern, states "that Allows for object level access control by acting as a pass through entity or a placeholder object. "
This pattern is recommended when,
- Objects need to be created on demand.
- Access control for the original object is required,
- Added functionality is required when an object is accessed.
Adapter design pattern is one of the structural design patterns and it allows two unrelated interfaces to work together. The object that joins these unrelated interface is called an Adapter.
The Adapter pattern is more about getting your existing code to work with a newer system or interface.
The Bridge pattern allows you to possibly have alternative implementations of an algorithm or system.
Adapter makes things work after they're designed; Bridge makes them work before they are.
The Chain of Responsibility is a behavioral pattern, which states that "Give more than one object an opportunity to handle a request by linking receiving objects together. "
In JavaEE, the Servlet filters implement the Chain of Responsibility pattern, and may also decorate the request to add extra information before the request is handled by a servlet.
Intercepting Filters trigger actions before or after an incoming request is processed by a handler.
Intercepting filters represents centralized components in a web application, common to all requests and extensible without affecting existing handlers.
AOP is based on Interception filter pattern.
We need to do subclassing just to instantiate a particular class.
Abstract factory provides better abstraction as it encapsulates a group of individual factories.
Singleton violates single responsibility principle.
Singleton classes cannot be subclassed.
They inherently cause code to be tightly coupled.
Any class that needs to be available to the entire application and only one instance should exist is the ideal candidate for becoming Singleton. One example of this is Runtime class that ensures only one Runtime environment available under JVM.
Double-checked locking prevents creating a duplicate instance of Singleton when call to getInstance() method is made in a multi-threading environment. In Double checked locking pattern as shown in below example, singleton instance is checked two times before initialization.
public class Singleton{ private static Singleton _INSTANCE; public static Singleton getInstance() { if(_INSTANCE==null) { synchronized(Singleton.class){ // double checked locking inside synchronized block if(_INSTANCE==null){_INSTANCE=new Singleton();} } } return _INSTANCE; } }
Do not implement the Cloneable interface at Singleton class and however if it implemented, override the clone method and throw an exception to prevent cloning.
You can prevent by using readResolve() method since during serialization readObject() is used to create an instance and it returns new instance every time. However, by using readResolve you can replace it with original Singleton instance.
Mediator design pattern is one of the behavioral design patterns and it is used to provide a centralized communication medium between different objects in a system.
Its intent is to "Allows loose coupling by encapsulating the way disparate sets of objects interact and communicate with each other. Allows for the actions of each object set to vary independently of one another".
- java.util.Timer class schedule...() method,
- Java Concurrency Executor execute() method.
- java.lang.reflect.Method invoke() method.
Command Pattern is one of the Behavioral Design Patterns and it is used to implement loose coupling in a request-response model.
java.lang.Runnable interface and Swing Action (javax.swing.Action) implements command design pattern.
State design pattern is one of the behavioral design patterns and it is used when an Object change its behavior based on its internal state.
If the cost of creating an object is larger and time consuming than the cost of cloning the object, then it is preferred to clone the object.
The Abstract Factory, Builder, and Prototype patterns may use singleton pattern. Also façade objects are often created using singleton pattern.
The Memento pattern is known as behavioural pattern and it "Captures and externalizes an object's internal state so that it can be restored later, all without violating encapsulation" .
The Memento pattern provides undo mechanism in your application when the internal state of an object need to be restored at a later stage. Using serialization along with this pattern, it's easy to preserve the object state and restore it later.
Visitor pattern is one of the behavioural patterns and it's definition is as follows, "allows for one or more operation to be applied to a set of objects at runtime, decoupling the operations from the object structure".
The value object pattern is an object that encapsulates a set of values that is moved across the boundary so that attempts to get the values of those attributes are local that eliminates redundant network calls. This pattern provides the best way to exchange data across tiers or system boundaries, especially when there is network communication involved. This is a pattern that solves performance issues around network latency.
Transfer object is a simple Java class with fields and contains no business logic. They are serializable POJO classes and have accessor methods (getter and setters) to access fields. These classes pass data between layers, they need not match business objects. Usually, Transfer objects group arguments to service method call.
Service Locator pattern is one of the core J2EE Design patterns.
Service Locator object abstracts JNDI usage and hides the complexities of initial context creation, EJB home object lookup, and EJB object re-creation. Multiple clients can reuse the Service Locator object to reduce code complexity, provide a single point of control, and improve performance by providing a caching facility.
At the Presentation layer, the most used design patterns are Intercepting filter, Front Controller, View Helper, Dispatcher View, Composite View, Application Controller and Context object.
At the Business Layer, the design patterns are Business Delegate, Session Facade, Transfer Object, Business Object, Value List Handler, Service Locator, Application Service and Transfer Object Assembler.
At the Integration Layer, the design patterns are DAO, Service Activator, Web service broker and Domain store.
Front Controller is a controller pattern which provides a centralized controller for managing requests. All incoming requests are delegated to front controller first and it is useful when your application has multiple entry points which you want to centralize through a single point for standardized processing.
View Helper, on the other hand, is in the view layer. It simply arranges view components for the user and delegates processing to other business components so the view component doesn't have to contain any processing logic other than logic to present views.Example is if you need a menu selection to persist through all the views, you store the menu items on the View Helper which calls different views but you will always have access to the menu. Usually both Front controller and view helper are used together.Factory Method Pattern allows the sub-classes to choose the type of objects to create.
Promotes the loose-coupling by eliminating the need to bind application-specific classes into the code. That means the code interacts solely with the resultant interface or abstract class, so that it will work with any classes that implement that interface or that extends that abstract class.
A monolithic architecture describes a single-tiered software application in which the user interface and data access code are combined into a single program from a single platform. For example, Java war file wraps UI component as well as controller and Business logic.
Yes. As per wiki,the template method is used in frameworks, where each implements the invariant parts of a domain's architecture, leaving "placeholders" for customisation options. This is an example for inversion of control, also called the Hollywood principle.
java.util.Collections.sort method implements template method pattern.
Additional overhead at garbage collection.
Constructor injection is complex which affects reflection based frameworks.
- Channel pattens,
- Routing patterns,
- Message construction patterns,
- Transformation and endpoint patterns.
A filter dynamically intercepts requests and responses to transform or use the information contained in the requests or responses. Filters typically do not themselves create responses, but instead provide universal functions that can be "attached" to any type of servlet or JSP page.
Interceptors are used in conjunction with Java EE managed classes to allow developers to invoke interceptor methods in conjunction with method invocations or lifecycle events on an associated target class. Common uses of interceptors are logging, auditing, or profiling.
Interceptors can be defined within a target class as an interceptor method, or in an associated class called an interceptor class. Interceptor classes contain methods that are invoked in conjunction with the methods or lifecycle events of the target class.