Java / Java Multithreading
Explain setUncaughtExceptionHandler method of Java Thread class.
The java.lang.Thread. setUncaughtExceptionHandler() method sets the handler to be invoked when this thread abruptly terminates due to an uncaught exception.
public void setUncaughtExceptionHandler(Thread.UncaughtExceptionHandler eh)
This method does not return any thing.
public class ExceptionHandlerEx { static class MyThreadClass implements Runnable { public void run() { throw new NullPointerException(); } } public static void main(String[] args) { Thread t = new Thread(new MyThreadClass()); t.setUncaughtExceptionHandler(new Thread.UncaughtExceptionHandler() { public void uncaughtException(Thread t, Throwable e) { System.out.println(t + " throws exception: " + e); } }); t.start(); } }
Dogecoin
! Earn free bitcoins up to $250 now by signing up.
Earn bitcoins upto $250 (free), invest in other Cryptocurrencies when you signup with blockfi.
Use the referral link: Signup now and earn!
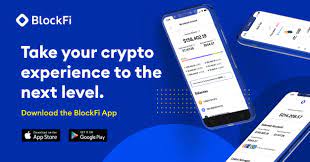
Using BlockFi, don't just buy crypto - start earning on it. Open an interest account with up to 8.6% APY, trade currencies, or borrow money without selling your assets.
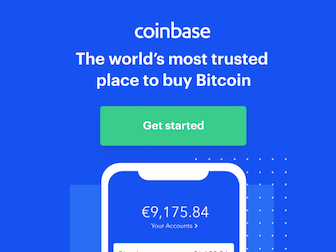
Join CoinBase
! We'll both receive $10 in free Bitcoin when they buy or sell their first $100 on Coinbase! Available in India also.
Use the referral Join coinbase!
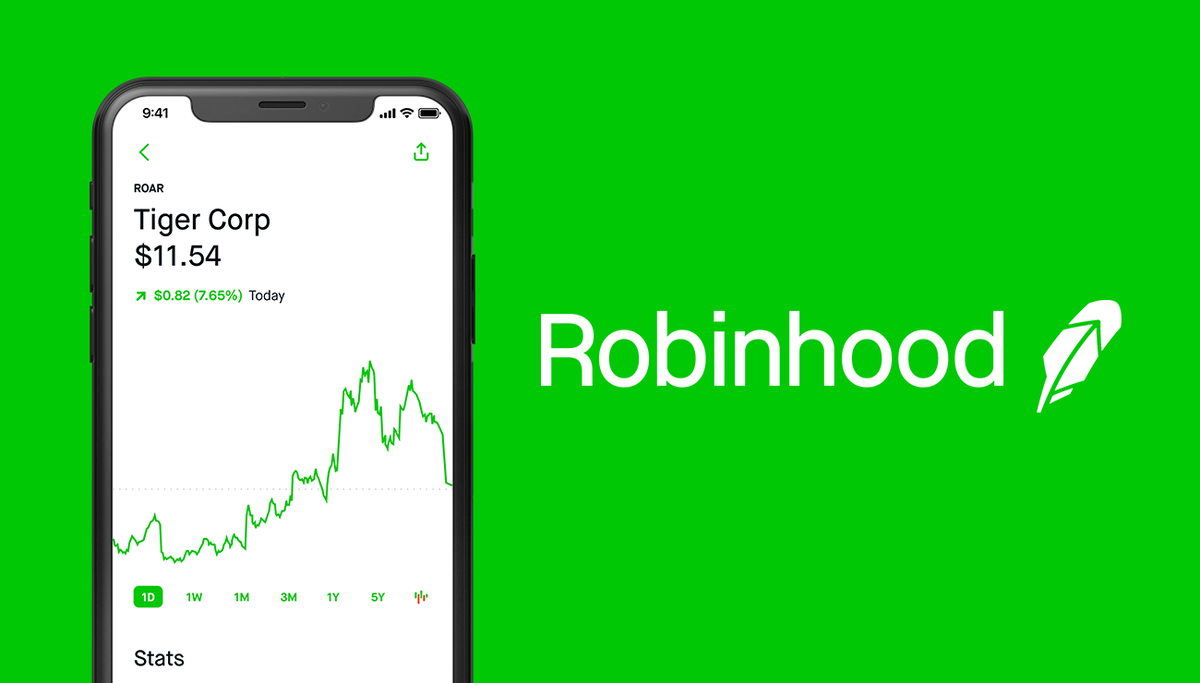
Invest now!!! Get Free equity stock (US, UK only)!
Use Robinhood app to invest in stocks. It is safe and secure. Use the Referral link to claim your free stock when you sign up!.
The Robinhood app makes it easy to trade stocks, crypto and more.
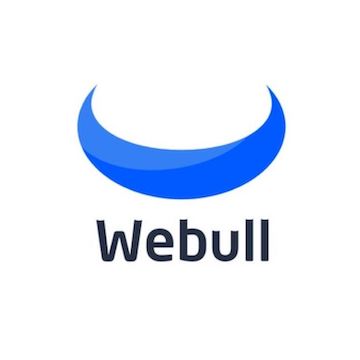
Webull
! Receive free stock by signing up using the link: Webull signup.
More Related questions...