Web / React native
React Native is an open-source framework for building Android and iOS applications using React and the app platform's native capabilities. With React Native, you use JavaScript to access your platform's APIs as well as to describe the appearance and behavior of your UI using React components: bundles of reusable, nestable code.
The concepts are based on react framework. React Native runs on React, a popular open-source library for building user interfaces with JavaScript.
Core Component include,
- <View>, a container that supports layout with flexbox, style, some touch handling, and accessibility controls.
- <Text>, displays, styles, and nests strings of text and even handles touch events.
- <Image>, displays various types of images.
- <ScrollView>, a generic scrolling container that can contain multiple components and views.
- <TextInput>, allows the user to enter text.
React and React Native use JSX, a syntax that lets you write elements inside JavaScript like so: <Text>Hello, I am your cat!</Text>.
JSX is a syntax extension to JavaScript and it produces React elements. It also renders variables and expressions.
Props is short for "properties". Props let you customize React components. For example, here you pass each <Cat> a different name for Cat to render:
import React from 'react'; import { Text, View } from 'react-native'; const Cat = (props) => { return ( <View> <Text>Hello, I am {props.name}!</Text> </View> ); } const Cafe = () => { return ( <View> <Cat name="Maru" /> <Cat name="Jellylorum" /> <Cat name="Spot" /> </View> ); }
State is useful for handling data that changes over time or that comes from user interaction. The state tracks your component variables and acts as private storage for your component.
Use props to configure a component when it renders. Use state to keep track of any component data that you expect to change over time.
Props are passed from a parent component, but the state is managed by the component itself. A component cannot change its props, but it can change its state.
Redux is a state management tool for JavaScript applications. You can use Redux together with React, or with any other view library.
It helps you write applications that behave consistently, run in different environments (client, server, and native), and are easy to test.
Redux is made up of the following key parts:
- actions,
- reducers,
- store,
- dispatch,
- selector.
Ducks is essentially a proposal for bundling reducers, action types, and actions of REDUX into the same file.
Hooks are the new feature introduced in the React 16.8 version. It allows you to use state and other React features without writing a class. Hooks are the functions which "hook into" React state and lifecycle features from function components.
useState and useEffect are few examples of React hooks.
The primary difference between Flux and Redux is that Flux uses multiple Stores per app, but Redux uses just one Store per app. Rather than placing state information in multiple Stores across the application, Redux keeps everything in one region of the app.
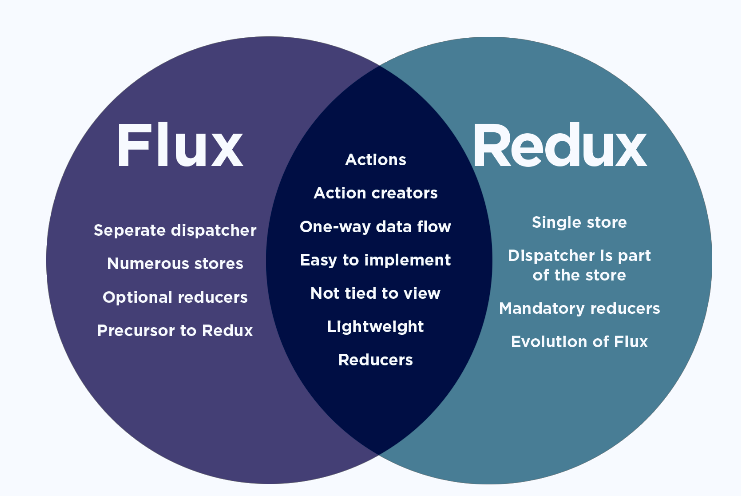
The main difference between useEffect hook and useLayoutEffect hook is in the timing of their invocation. useEffect hook is invoked after the DOM is painted. useLayoutEffect hook on the other hand is invoked synchronously before changes are painted on the screen.
Use useLayoutEffect hook instead of useEffect hook if your effect will mutate the DOM. useEffect hook is called after the screen is painted. Therefore mutating the DOM again immediately after the screen has been painted, will cause a flickering effect if the mutation is visible to the client.
Native mobile apps are the most common type of apps that are built for specific platforms and are written in languages that is platform specific. For example, Swift and Objective-C for native iOS apps and Java or Kotlin for native Android apps.
Native apps are also built using the specific Integrated Development Environment (IDE) for the selected operating systems such as Android Studio and Xcode for IOS.
- Native Apps have the best performance.
- Native Apps are more Secure.
- Native Apps are more Interactive And Intuitive.
- Native Apps allow developers to access the full feature set of devices.
- Native App development tends to have fewer bugs.
Offline first is an application development paradigm where developers ensure that the functionality of an app is unaffected by the intermittent lack of a network connection. In addition to that, "offline first" usually implies the ability to sync data between multiple devices.
JavaScript Obfuscator converts or encodes the actual source code of JavaScript to an unreadable format by making the code into machine-level language because stealing the code from the unauthorized end user. This process is 100% safe in JavaScript and the best way to protect source code.
Component-Driven Development (CDD) is a development methodology that anchors the build process around components. It is a process that builds UIs from the "bottom-up" by starting at the level of components and ending at the level of pages or screens.
In this methodology, we split the complete UI design into several small reusable components and develop them independently, and the complete UI is built by composing these small components.
React Native uses watchman to detect when you've made code changes and then automatically build and push the update your device without you needing to manually refresh it.
The Facebook watchman service is designed to scale to very large filesystem trees and to aggregate watching resources across multiple projects.
Refs provide a way to access DOM nodes or React elements created in the render method.
There are a few good use cases for refs:
- Managing focus, text selection, or media playback.
- Triggering imperative animations.
- Integrating with third-party DOM libraries.
The default value for position property is relative whereas in browser it is static.
There are 2 ways of writing a react component.
Functional component uses a simple function which returns the JSX.
Class based component uses the class keyword introduced in ES6. it implements render lifecycle method which returns the JSX.
Functional component. | Class-based component. |
Functional component cannot have state ( before React hooks, now it can have state with React 16.8 or higher). It renders component only based on props that are supplied from the parent. | A class-based component can have component level state. |
Functional component are simple functions and light weighted. These are functions that accepts props as an argument and returns a React element. | A class-based component is a complex structure. It is an instance of a class derived from React. Component class. The class must implement a render() member function which returns a React component to be rendered. |
functional component cannot use lifecycle methods. | The class-based component use lifecycle methods such as componentDidMount. |
Functional component example:
function Hello(props) { return <h1>Hello, {props.userName}</h1>; }
Class based component example:
class Hello extends React.Component { render() { return <h1>Hello, {this.props.userName}</h1>; } }
The useFocusEffect is analogous to React's useEffect hook. The only difference is that it only runs if the screen is currently focused.
The useFocusEffect hook runs the effect as soon as the screen comes into focus. The effect will run whenever the dependencies passed to React.useCallback change, i.e. it'll run on the initial render (if the screen is focused) as well as on subsequent renders if the dependencies have changed.
React Navigation is a standalone library that enables you to implement navigation functionality in a React Native application. It also enables structuring your application using multiple screens.
React Navigation is written in JavaScript and does not directly use the native navigation APIs on iOS and Android.
The 2 axes are main axis and the cross axis. The main axis is defined by the flex-direction property, and the cross axis runs perpendicular to it.
The main axis is defined by flex-direction, which has four possible values: row, row-reverse, column, column-reverse. For row or row-reverse, your main axis will run along the row in the inline direction (row- left to right, row-reverse-right to left). For column or column-reverse it is block direction.
The cross axis runs perpendicular to the main axis, therefore if your flex-direction (main axis) is set to row or row-reverse the cross axis runs down the columns. If your main axis is column or column-reverse then the cross axis runs along the rows.
marginVertical is not part of standard CSS but with react-native, setting marginVertical has the same effect as setting both marginTop and marginBottom.
ScrollView renders all its react child components at once, but this has a performance downside.
FlatList renders items lazily, when they are about to appear, and removes items that scroll way off-screen to save memory and processing time.
Single source of truth - the global state of your application is stored in an object tree within a single store.
State is read-only - the only way to change the state is to emit an action, an object describing what happened.
Changes are made with pure function - To specify how the state tree is transformed by actions, write pure reducers.
- Stack Navigator,
- Native Stack Navigator,
- Drawer Navigator,
- Bottom Tab Navigator,
- Material Bottom Tab Navigator,∫li>
- Material Top Tab Navigator.
React Navigation's stack navigator provides a way for your app to transition between screens and manage navigation history.
Stack navigator provides a way for your app to transition between screens where each new screen is placed on top of a stack.
ReactJS is a JavaScript library, supporting both front-end web and being run on a server, for building user interfaces and web applications. It follows the concept of reusable components.
React Native is a mobile framework that makes use of the JavaScript engine available on the host, allowing you to build mobile applications for different platforms (iOS, Android, and Windows Mobile) in JavaScript that allows you to use ReactJS to build reusable components and communicate with native components.
Both follow the JSX/TSX syntax extension of JavaScript.
React native elements is a Cross Platform UI Toolkit for React Native. It is cross-platform across android, IOS and web.
Built completely in Javascript.
- Slow startup time,
- Time to first interaction,
- Animation jagged/slow,
- and slow response to user action.
React Native bridge is a C++/Java bridge which is responsible for communication between the native and Javascript thread. A custom protocol is used for message passing.
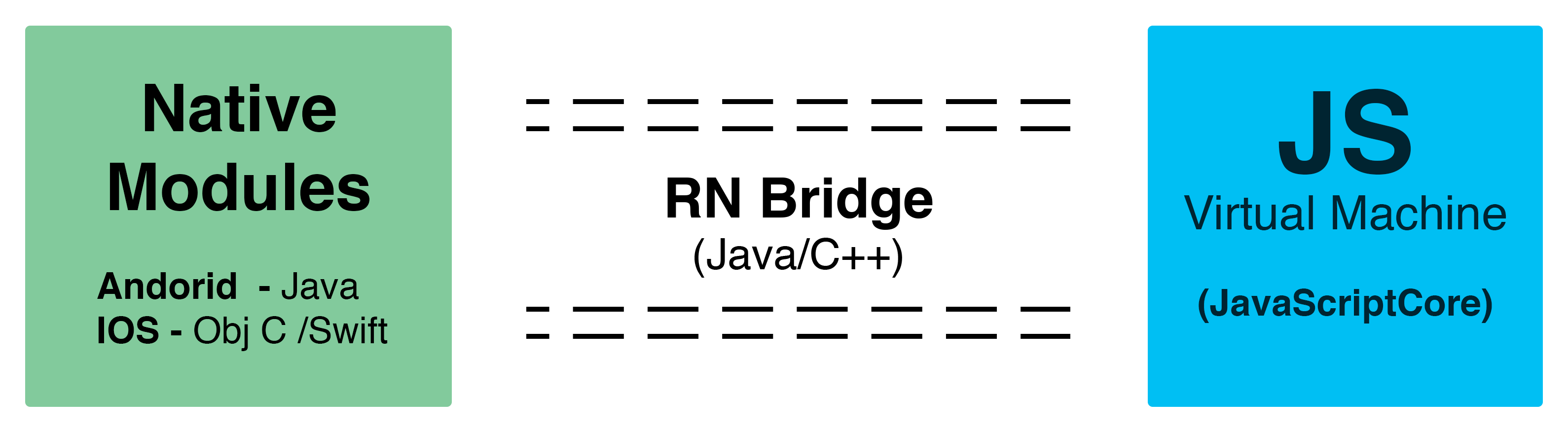
React native uses 3 threads,
- MAIN/UI Thread, main application thread on which your Android/iOS app is running. The UI of the application can be changed by the Main thread.
- Shadow Thread, layout created using React library in React Native can be calculated by this and it is a background thread.
- JavaScript Thread, Javascript code is executed by this thread.
Redux is a predictable state container for JavaScript apps. It helps write applications that run in different environments. This means the entire data flow of the app is handled within a single container while persisting in the previous state.
Declaring a function outside of the class is like making a static function. If you place the function in the class, it will be created for each instance of the class which in this case would be unnecessary.
Yes.When an action is dispatched, useSelector() will do a reference comparison of the previous selector result value and the current result value. If they are different, the component will be forced to re-render. If they are the same, the component will not re-render.
React components automatically re-render whenever there is a change in their state or props. State modification, passing props, using the Context API, passing references(array, function, and object) causes rerenders.
if the parent component has triggered a rerender all the child component will rerender too, it does not matter if the props are consumed, modified or not (at the child components), the child components will re-render if the parent component has triggered a render.
index.js is the first file that loads which in turn loads App component.
ReactJS knowledge can be leveraged for React-native however there few differences.
ReactJS is for developing web applications while React-native developing mobile applications.
We can use HTML tags in ReactJS however react native doesn't understand html tags.
In ReactJS, the virtual DOM renders the browser code, while React-native uses its API to render code for mobile applications.
ReactJS uses React-router for navigating web pages however react-native uses a built-in navigator library (React-navigation) for navigating mobile applications.
It is a layout model that allows elements to align and distribute space within a container. With Flexbox when Using flexible widths and heights, all the inside the main container can be aligned to fill a space or distribute space between elements, which makes it a great tool to use for responsive design systems.
To achieve the desired layout, flexbox offers three main properties: flexDirection, justifyContent and alignItems. The following table shows the possible options.
Property | Values | Description |
flexDirection | 'column', 'row' | Used to specify if elements will be aligned vertically or horizontally. |
justifyContent | 'center', 'flex-start', 'flex-end', 'space-around', 'space-between' | Used to determine how should elements be distributed inside the container. |
alignItems | 'center', 'flex-start', 'flex-end', 'stretched' | Used to determine how should elements be distributed inside the container along the secondary axis (opposite of flexDirection). |
It is used to control the components. The variable data can be stored in the state. It is mutable means a state can change the value at any time.
const HomeScreen = React.memo(({ navigation }) => { console.log('HomeScreen rendered'); const [myState, setMyState] = useState("Test Value"); const updateState = () => { setMyState('The state is updated') } return ( <View> <Text onPress={updateState}> {myState} </Text> </View> ); });
Flipper is a platform for debugging iOS, Android, and React Native apps. Visualize, inspect, and control your apps from a simple desktop interface.
Redux persist provides general redux state persistence, while redux-offline solves the high order problem of managing offline effects.
Both redux persist and redux offline persist state, In addition, redux-offline manages offline effects (retry + commit + rollback)(actions being performed when offline).
Redux Offline uses the redux-persist library.
Each component in React has a lifecycle which you can monitor and manipulate during its three main phases. The 3 phases are: Mounting, Updating, and Unmounting.
During Mounting phase, 4 methods gets called in the below order.
- constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
The render() method is mandatory and will be always called, while others are optional.
constructor()
method is called before anything else, when the component is initiated, and it is the place to set up the initial state and other initial values.
The constructor() method is called with the props, as arguments, and you should always start by calling the super(props) before anything else, this will initiate the parent's constructor method and allows the component to inherit methods from its parent (React.Component).
The getDerivedStateFromProps()
method is called right before rendering the element(s) in the DOM.It is the place to set the state object based on the initial props.It takes state as an argument, and returns an object with changes to the state.
The render()
method is required, and is the method that actually outputs the HTML to the DOM.
componentDidMount
is executed after the first render only on the client side. This is where AJAX requests and DOM or state updates should occur. This method is also used for integration with other JavaScript frameworks and any functions with delayed execution such as setTimeout or setInterval. We are using it to update the state so we can trigger the other lifecycle methods.
Update Phase: A component is updated whenever there is a change in the component's state or props. 5 built-in methods are called in the below order when a component is updated.
- getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
All methods except render are optional and will be called if you define it.
Also at updates the getDerivedStateFromProps
method is called. This is the first method that is called when a component gets updated.
This is still the place to set the state object based on the initial props.
In the getSnapshotBeforeUpdate()
method you have access to the props and state before the update, meaning that even after the update, you can check what the values were before the update.
If the getSnapshotBeforeUpdate() method is present, you should also include the componentDidUpdate() method, otherwise you will get an error.
shouldComponentUpdate
should return a true or false value. This will determine if the component will be updated or not. This is set to true by default. If you are sure that the component doesn't need to render after state or props are updated, you can return a false value.
componentDidUpdate
is called just after rendering.
Deprecated/Unpopular lifecycle methods:
componentWillUnmount
is called after the component is unmounted from the dom. We are unmounting our component in main.js.
componentWillMount
is executed before rendering, on both the server and the client-side.This method is only called one time, which is before the initial render.
componentWillReceiveProps
is invoked as soon as the props are updated before another render is called. We triggered it from setNewNumber when we updated the state.
componentWillUpdate
is called just before rendering.
We may want to run side-effects when a screen is focused such as adding an event listener, fetching data, updating document title, etc.
useFocusEffect executes every time when the screen is focused. The useFocusEffect is analogous to React's useEffect hook. The only difference is that it only runs if the screen is currently focused.
import { useFocusEffect } from '@react-navigation/native'; function Profile({ userId }) { const [user, setUser] = React.useState(null); useFocusEffect( React.useCallback(() => { const unsubscribe = API.subscribe(userId, user => setUser(user)); return () => unsubscribe(); }, [userId]) ); return <ProfileContent user={user} />; }
Redux middleware is a function or a piece of code that sits between action and reducer and can interact with the dispatched action before reaching the reducer function.
A redux middleware can be used for many purposes such as logging (for example, redux-logger), asynchronous API calls, and so on.
Redux Thunk and Redux Saga are some of the popular redux middlewares.
The purpose of SafeAreaView is to render content within the safe area boundaries of a device. It is currently only applicable to iOS devices with iOS version 11 or later.
useLayoutEffect is for sync update and useEffect is for async updates. useEffect runs async while first render being performed, useLayoutEffect runs before first render.
You can dispatch navigation actions from the navigation container where you do not have access to the navigation prop, such as a Redux middleware.
To navigate from inside a component without needing to pass the navigation prop down, you may use useNavigation hook.
Code Quality: By moving styles away from the render function, you're making the code easier to understand. Naming the styles is a good way to add meaning to the low level components in the render function.
Performance: Making a stylesheet from a style object makes it possible to refer to it by ID instead of creating a new style object every time. It also allows sending the style only once through the bridge. All subsequent uses are going to refer an id (not implemented yet).