Java / JavaFX
JavaFX is a set of graphics and media packages that enables developers to design, create, test, debug, and deploy rich client applications that operate consistently across diverse platforms.
It can be considered as an alternative to Java Swing.
Yes. As part of JDK 1.9 it is deprecated.
A javafx.stage.Stage
in JavaFX is a top-level container that hosts a Scene, which consists of visual elements. The platform creates the primary stage and pass it to the start(Stage s)
method of the Application class.
The main class for a JavaFX application extends the javafx.application.Application
class.
The start
() method is the main entry point for all JavaFX applications. So your main class will override it.
A JavaFX application defines the user interface container by means of a stage and a scene. The JavaFX Stage
class is the top-level JavaFX container. The JavaFX Scene
class is the container for all content.
In JavaFX, the content of the scene is represented as a hierarchical scene graph of nodes.
The main() method is not required for JavaFX application, however useful to launch in IDE without JavaFX launcher.
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class Main extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("Welcome to Javapedia.net"); Button b = new Button("Click"); StackPane layout = new StackPane(); layout.getChildren().add(b); Scene scene = new Scene(layout, 300, 300); primaryStage.setScene(scene); primaryStage.show(); } }
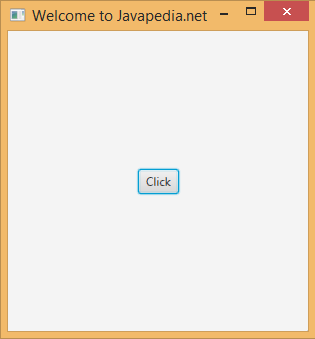
- BorderPane,
- HBox,
- VBox,
- StackPane,
- GridPane,
- FlowPane,
- TilePane,
- and AnchorPane.
javafx.stage holds the top level container classes for JavaFX application.
javafx.scene provides classes and interfaces to support the scene graph. In addition, it also provides sub-packages such as canvas, chart, control, effect, image, input, layout, media, paint, shape, text, transform, web, etc. There are several components that support this rich API of JavaFX.
javafx.animation has classes to add transition based animations such as fill, fade, rotate, scale and translation, to the JavaFX nodes.
javafx.application contains a set of classes responsible for the JavaFX application life cycle.
javafx.css contains classes to add CSS–like styling to JavaFX GUI applications.
javafx.event contains classes and interfaces to deliver and handle JavaFX events.
javafx.geometry contains classes to define 2D objects and perform operations on them.