Hibernate / Hibernate Interview Questions III
For web applications, it is ideal to have the servlet container manage the connection pool. This is achieved by defining JNDI resource for DataSource and use it in the web application. Use the below property to set the JNDI DataSource name.
<property name="hibernate.connection.datasource">java:comp/env/jdbc/MyDBJNDI</property>
There are different ways to implement joins in Hibernate.
- Using associations such as one-to-one, one-to-many,
- Using JOIN in the HQL query,
- and by using native sql query with join keyword.
- Load configuration file and create instance of configuration class.
- Using configuration object, create SessionFactory object.
- From SessionFactory, create session.
- Create HQL query.
- Execute HQL query and get the results.
Cascading consists in propagating the Parent entity state transition to one or more Child entities, and it can be used for both unidirectional and bidirectional associations.
Using Criteria addQueryHint method, we can specify database specific hint.
Criteria addQueryHint(String hint)
In JDBC positional parameter index starts at 1, while it starts at 0 in Hibernate create sql query.
@Formula annotation is used to calculate a given entity attribute using an SQL query expression. It defines a formula (derived value) which is a SQL fragment that acts as a @Column alternative in most cases. It represents read-only state.
@Entity @Table(name="area") public class Area implements Serializable { private static final long serialVersionUID = 1L; @Id @Column(name="id") private int id; @Column(name="length") private int length; @Column(name="width") private int width; @Formula(" length * width ") public long area; ... }
In the example, area property value is calculated using the length and the width properties.
Use Query.setMaxResults() to limit the result.
You can define an ORDER BY clause with the orderBy method of the CriteriaQuery interface and the asc or desc method of the CriteriaBuilder interface.
CriteriaBuilder cb = em.getCriteriaBuilder(); CriteriaQuery<Employee> cq = cb.createQuery(Employee.class); Root<Book> root = cq.from(Employee.class); cq.orderBy(cb.asc(root.get(Employee_.firstName))); // Execute query with pagination List<Employee> emplList = em.createQuery(cq).getResultList();
The life cycle of entity objects consists of four states: New, Managed, Removed and Detached.
When an entity object is initially created its state is New. In this state the object is not yet associated with an EntityManager and has no representation in the database.
An entity object becomes Managed when it is persisted to the database via an EntityManager's persist method, which must be invoked within an active transaction.
A managed entity object can also be retrieved from the database and moved to removed, by using the EntityManager's remove method within an active transaction.
Detached represents entity objects that have been disconnected from the EntityManager.
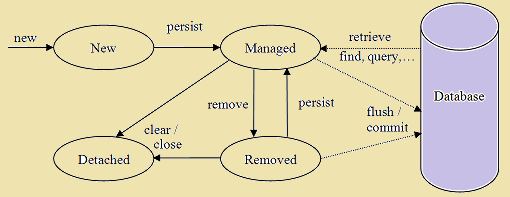
Java Applications and components are managed in hibernate by a standard API called JMX API. JMX provides tools for development of efficient and robust distributed, web based solutions.
Yes, hibernate fully supports polymorphism. Polymorphism queries and polymorphism associations are supported in all mapping strategies of hibernate.
- Identify natural keys for all entities, and map them.
- Place each class mapping in its own file.
- Externalize query strings to make applications more portable.
- Use bind variables to prevent SQL Injection.
- Use hand-coded JDBC sparing: Using SQL defeats the entire purpose of using Hibernate. So, use it sparingly, only when it is performance-critical.
- Prefer lazy fetching for associations. Explicitly disable eager fetching using lazy="false". When join fetching is appropriate to a particular use case, use a query with a left join fetch.
- Use bidirectional associations: In a large application, almost all associations must be navigable in both directions in queries.
Transient objects do not have association with the database and session objects. They are simple objects and not persisted to the database. Once the last reference is lost, that means the object itself is lost. And of course , garbage collected.
The detached object have corresponding entries in the database. These are persistent and not connected to the Session object. These objects have the synchronized data with the database when the session is closed.
It has no effect and the transient object retains its state.
There is no relationship between cascade and inverse, both serves different purpose.
It defines which side is the parent or the relationship owner for the two entities. Hence, inverse="true" in a Hibernate mapping shows that this designated class is the relationship owner; while the other class is the child.
The JPA @ManyToOne and @OneToOne annotations are fetched EAGERly by default, while the @OneToMany and @ManyToMany relationships are LAZY by default.
The attribute mappedBy indicates that the entity in this side is the inverse of the relationship, and the owner resides in the "other" entity. This also means that you can access the other table from the class which you've annotated with "mappedBy" (fully bidirectional relationship).
@Entity public class Movie { @Id @GeneratedValue(strategy = GenerationType.AUTO) @Column(name = "id", updatable = false, nullable = false) private Long id; @OneToMany(mappedBy = "movie") private List reviews = new ArrayList(); .. }
b = entityManager.find(Movie.class, 122L); List reviews = b.getReviews(); Assert.assertEquals(b, reviews.get(0).getMovie());
mappedBy. | joinColumn. |
The attribute mappedBy indicates that the entity in this side is the inverse of the relationship, and the owner resides in the "other" entity. | The annotation @JoinColumn indicates that the entity is the owner of the relationship. This means that the corresponding table has a column with a foreign key to the referenced table. |
You can access the other table from the class which you have annotated with "mappedBy" (fully bidirectional relationship). | You cannot access other table and its one directional. |